Lagrangian particle tracking with ACCESS-OM2-01.¶
Lagrangian particle tracking is widely used in a range of physical and biological oceanography applications. This review paper on Lagrangian fundamentals covers many of the basics and provides an overview of the different particle tracking software programs available to the oceanography community. In this notebook we’ll use Parcels which has the advantage of a large user community and an active development team. The Parcels website is well documented and has some great general tutorials to get you started. In this tutorial, we’ll focus on how to get a simple particle tracking experiment running with output from the ACCESS-OM2-01 model.
Requirements: conda/analysis3-23.07
(or later)
Firstly, load in the required modules.
[1]:
import numpy as np
import xarray as xr
import matplotlib as mpl
import matplotlib.pyplot as plt
import cosima_cookbook as cc
from glob import glob
from datetime import timedelta
from dask.distributed import Client
from parcels import FieldSet, ParticleSet, Variable, JITParticle, ScipyParticle, AdvectionRK4, AdvectionRK4_3D, __version__
[2]:
print('parcels', __version__)
parcels 3.0.0
It’s often a good idea to start a cluster with multiple cores for you to work with.
[3]:
client = Client(n_workers=4)
client
[3]:
Client
Client-33936c14-7c33-11ee-8fa8-00000084fe80
Connection method: Cluster object | Cluster type: distributed.LocalCluster |
Dashboard: /proxy/8787/status |
Cluster Info
LocalCluster
ab16377a
Dashboard: /proxy/8787/status | Workers: 4 |
Total threads: 12 | Total memory: 46.00 GiB |
Status: running | Using processes: True |
Scheduler Info
Scheduler
Scheduler-c61aa733-6d4e-4a60-be37-c69748bf7295
Comm: tcp://127.0.0.1:44369 | Workers: 4 |
Dashboard: /proxy/8787/status | Total threads: 12 |
Started: Just now | Total memory: 46.00 GiB |
Workers
Worker: 0
Comm: tcp://127.0.0.1:42305 | Total threads: 3 |
Dashboard: /proxy/45567/status | Memory: 11.50 GiB |
Nanny: tcp://127.0.0.1:40265 | |
Local directory: /jobfs/99990320.gadi-pbs/dask-scratch-space/worker-5ukzjpcj |
Worker: 1
Comm: tcp://127.0.0.1:42627 | Total threads: 3 |
Dashboard: /proxy/33055/status | Memory: 11.50 GiB |
Nanny: tcp://127.0.0.1:34599 | |
Local directory: /jobfs/99990320.gadi-pbs/dask-scratch-space/worker-ahho_u_b |
Worker: 2
Comm: tcp://127.0.0.1:35631 | Total threads: 3 |
Dashboard: /proxy/45535/status | Memory: 11.50 GiB |
Nanny: tcp://127.0.0.1:43505 | |
Local directory: /jobfs/99990320.gadi-pbs/dask-scratch-space/worker-cd1z26uw |
Worker: 3
Comm: tcp://127.0.0.1:32847 | Total threads: 3 |
Dashboard: /proxy/45459/status | Memory: 11.50 GiB |
Nanny: tcp://127.0.0.1:43133 | |
Local directory: /jobfs/99990320.gadi-pbs/dask-scratch-space/worker-0zbrqc_l |
Define the velocity fields and any other model data we want Parcels to read
We’re now going to run some simple offline Lagrangian particle tracking experiments. This means we need to read in some velocity fields that have been output from an ocean model and feed these to Parcels. Generally speaking, you want to run Lagrangian particle tracking experiments with the highest temporal resolution available. We have several periods of daily output from ACCESS-OM2-01 available on Gadi. These are listed below.
21 years of daily
u,v,wt
from the RYF simulation corresponding to model years 50-71. This is stored in foldersoutput146-output279
here:/g/data/ik11/outputs/access-om2-01/01deg_jra55v13_ryf9091/
31 years of daily
u,v,wt
from cycle 1 of the IAF simulation from 1987 to 2018. This is stored in foldersoutput116 - output243
here:/g/data/cj50/access-om2/raw-output/access-om2-01/01deg_jra55v140_iaf
We’ll use the first year of the daily RYF data for this experiment. We’ll also start with a simple 2D advection example, and then run a 3D particle advection experiment with temperature and salinity.
[4]:
dir = ! echo /scratch/$PROJECT/$USER/particle_tracking
print(f"WARNING: Output is being saved in this directory: {dir[0]} \nChange to any directory you would like.")
output_directory = dir[0]
output_directory
WARNING: Output is being saved in this directory: /scratch/tm70/as2285/particle_tracking
Change to any directory you would like.
[4]:
'/scratch/tm70/as2285/particle_tracking'
2D Advection¶
[5]:
%%time
# First, we need to define ACCESS-OM2-01 (MOM5 ocean) NetCDF files.
data_path = '/g/data/ik11/outputs/access-om2-01/01deg_jra55v13_ryf9091/'
# At a minimum, Parcels needs U and V files (2D advection).
ufiles = sorted(glob(data_path+'output19*/ocean/ocean_daily_3d_u_*.nc'))
vfiles = sorted(glob(data_path+'output19*/ocean/ocean_daily_3d_v_*.nc'))
# We then need to read in a file that contains the grid coordinates which are saved in the `ocean.nc` file.
# However our daily velocity data is only saved up to -32.59S and does not cover the whole globe.
# We need to select out only the Southern Ocean region and resave the grid coordinates to file.
orig_mesh_mask = '/g/data/ik11/outputs/access-om2-01/01deg_jra55v13_ryf9091/output196/ocean/ocean.nc'
ds = xr.open_dataset(orig_mesh_mask).sel(yu_ocean=slice(None, -32.52)).sel(yt_ocean=slice(None, -32.55))
variables = ['v', 'wt']
ds = ds[variables]
mesh_mask = output_directory+'ocean.nc'
ds.to_netcdf(mesh_mask)
CPU times: user 2min 29s, sys: 11.7 s, total: 2min 41s
Wall time: 2min 40s
[6]:
# We now define a dictionary that tells Parcels where the U,V data is, and where to
# find the coordinates.
filenames = {
'U': {'lon': mesh_mask, 'lat': mesh_mask, 'depth': mesh_mask, 'data': ufiles},
'V': {'lon': mesh_mask, 'lat': mesh_mask, 'depth': mesh_mask, 'data': vfiles},
}
# Now define a dictionary that specifies the `U, V` variable names as given in the netcdf files
variables = { 'U': 'u', 'V': 'v'}
# Define a dictionary to specify the U,V dimentions.
# See the description under the 3D advection section for why we specify sw_ocean as the depth here.
# For further reading, also see this tutorial on the Ocean Parcels website: https://nbviewer.org/github/OceanParcels/parcels/blob/master/parcels/examples/documentation_indexing.ipynb
dimensions = {
'U': {'lon': 'xu_ocean', 'lat': 'yu_ocean', 'depth': 'sw_ocean', 'time': 'time'},
'V': {'lon': 'xu_ocean', 'lat': 'yu_ocean', 'depth': 'sw_ocean', 'time': 'time'}
}
indices = {'sw_ocean': range(0, 3)}
# Define the chunksizes for each variable. This will help Parcels read in the FieldSet.
cs = {
"U": {"lon": ("xu_ocean", 400), "lat": ("yu_ocean", 300), "depth": ("st_ocean", 7), "time": ("time", 1)},
"V": {"lon": ("xu_ocean", 400), "lat": ("yu_ocean", 300), "depth": ("st_ocean", 7), "time": ("time", 1)},
}
# Finally, we load the fieldset using the Parcels `FieldSet.from_mom5` function.
fieldset = FieldSet.from_mom5(filenames, variables, dimensions, indices,
mesh = 'spherical',
chunksize = cs,
tracer_interp_method = 'bgrid_tracer')
We need to tell Parcels that this fieldset is periodic in the zonal (east-west) direction. This is because, if the particle is close to the edge of the fieldset (but still in it), the advection scheme will need to interpolate velocities that may lay outside the fieldset domain. With the halo, we make sure the advection kernel can access these values.
[7]:
fieldset.add_constant('halo_west', fieldset.U.grid.lon[0])
fieldset.add_constant('halo_east', fieldset.U.grid.lon[-1])
fieldset.add_periodic_halo(zonal=True)
WARNING: The zonal halo is located at the east and west of current grid, with a dx = lon[1]-lon[0] between the last nodes of the original grid and the first ones of the halo. In your grid, lon[1]-lon[0] != lon[-1]-lon[-2]. Is the halo computed as you expect?
We now need a custom kernel that can move the particle from one side of the domain to the other.
[8]:
def periodicBC(particle, fieldset, time):
if particle.lon < fieldset.halo_west:
particle_dlon += fieldset.halo_east - fieldset.halo_west
elif particle.lon > fieldset.halo_east:
particle_dlon -= fieldset.halo_east - fieldset.halo_west
We’ll also add a recovery kernel to delete particles if they encounter the ErrorOutOfBounds
signal from Parcels.
[9]:
def checkOutOfBounds(particle, fieldset, time):
if particle.state == StatusCode.ErrorOutOfBounds:
particle.delete()
Now we can create the Particle Set. This contains the particle starting locations and type of particle. We’ll read in the ht
field from the model and initialise particles in a small section across the ACC.
[10]:
session = cc.database.create_session()
experiment = '01deg_jra55v13_ryf9091'
ht = cc.querying.getvar(experiment, 'ht', session, n=1).load()
[11]:
x1, x2 = -100.5, -100
y1, y2 = -60, -65
lons = ht.where((ht.yt_ocean<y1)&(ht.yt_ocean>y2)&(ht.xt_ocean<x2)&(ht.xt_ocean>x1),
drop=True).geolon_t.values.ravel()
lats = ht.where((ht.yt_ocean<y1)&(ht.yt_ocean>y2)&(ht.xt_ocean<x2)&(ht.xt_ocean>x1),
drop=True).geolat_t.values.ravel()
depths = np.full(len(lats), 1.)
pset = ParticleSet.from_list(fieldset=fieldset, # the fields on which the particles are advected
pclass=JITParticle, # the type of particles (JITParticle or ScipyParticle)
lon=lons, # a vector of release longitudes
lat=lats, # a vector of release latitudes
depth=depths, # a vector of release depths
time=0.) # set time to start start time of daily velocity fields
pset
[11]:
P[0](lon=-100.449997, lat=-64.973167, depth=1.000000, time=0.000000)
P[1](lon=-100.349998, lat=-64.973167, depth=1.000000, time=0.000000)
P[2](lon=-100.250000, lat=-64.973167, depth=1.000000, time=0.000000)
P[3](lon=-100.150002, lat=-64.973167, depth=1.000000, time=0.000000)
P[4](lon=-100.050003, lat=-64.973167, depth=1.000000, time=0.000000)
P[5](lon=-100.449997, lat=-64.930832, depth=1.000000, time=0.000000)
P[6](lon=-100.349998, lat=-64.930832, depth=1.000000, time=0.000000)
P[7](lon=-100.250000, lat=-64.930832, depth=1.000000, time=0.000000)
P[8](lon=-100.150002, lat=-64.930832, depth=1.000000, time=0.000000)
P[9](lon=-100.050003, lat=-64.930832, depth=1.000000, time=0.000000)
P[10](lon=-100.449997, lat=-64.888428, depth=1.000000, time=0.000000)
P[11](lon=-100.349998, lat=-64.888428, depth=1.000000, time=0.000000)
P[12](lon=-100.250000, lat=-64.888428, depth=1.000000, time=0.000000)
P[13](lon=-100.150002, lat=-64.888428, depth=1.000000, time=0.000000)
P[14](lon=-100.050003, lat=-64.888428, depth=1.000000, time=0.000000)
P[15](lon=-100.449997, lat=-64.845955, depth=1.000000, time=0.000000)
P[16](lon=-100.349998, lat=-64.845955, depth=1.000000, time=0.000000)
P[17](lon=-100.250000, lat=-64.845955, depth=1.000000, time=0.000000)
P[18](lon=-100.150002, lat=-64.845955, depth=1.000000, time=0.000000)
P[19](lon=-100.050003, lat=-64.845955, depth=1.000000, time=0.000000)
P[20](lon=-100.449997, lat=-64.803413, depth=1.000000, time=0.000000)
P[21](lon=-100.349998, lat=-64.803413, depth=1.000000, time=0.000000)
P[22](lon=-100.250000, lat=-64.803413, depth=1.000000, time=0.000000)
P[23](lon=-100.150002, lat=-64.803413, depth=1.000000, time=0.000000)
P[24](lon=-100.050003, lat=-64.803413, depth=1.000000, time=0.000000)
P[25](lon=-100.449997, lat=-64.760811, depth=1.000000, time=0.000000)
P[26](lon=-100.349998, lat=-64.760811, depth=1.000000, time=0.000000)
P[27](lon=-100.250000, lat=-64.760811, depth=1.000000, time=0.000000)
P[28](lon=-100.150002, lat=-64.760811, depth=1.000000, time=0.000000)
P[29](lon=-100.050003, lat=-64.760811, depth=1.000000, time=0.000000)
P[30](lon=-100.449997, lat=-64.718132, depth=1.000000, time=0.000000)
P[31](lon=-100.349998, lat=-64.718132, depth=1.000000, time=0.000000)
P[32](lon=-100.250000, lat=-64.718132, depth=1.000000, time=0.000000)
P[33](lon=-100.150002, lat=-64.718132, depth=1.000000, time=0.000000)
P[34](lon=-100.050003, lat=-64.718132, depth=1.000000, time=0.000000)
P[35](lon=-100.449997, lat=-64.675392, depth=1.000000, time=0.000000)
P[36](lon=-100.349998, lat=-64.675392, depth=1.000000, time=0.000000)
P[37](lon=-100.250000, lat=-64.675392, depth=1.000000, time=0.000000)
P[38](lon=-100.150002, lat=-64.675392, depth=1.000000, time=0.000000)
P[39](lon=-100.050003, lat=-64.675392, depth=1.000000, time=0.000000)
P[40](lon=-100.449997, lat=-64.632584, depth=1.000000, time=0.000000)
P[41](lon=-100.349998, lat=-64.632584, depth=1.000000, time=0.000000)
P[42](lon=-100.250000, lat=-64.632584, depth=1.000000, time=0.000000)
P[43](lon=-100.150002, lat=-64.632584, depth=1.000000, time=0.000000)
P[44](lon=-100.050003, lat=-64.632584, depth=1.000000, time=0.000000)
P[45](lon=-100.449997, lat=-64.589706, depth=1.000000, time=0.000000)
P[46](lon=-100.349998, lat=-64.589706, depth=1.000000, time=0.000000)
P[47](lon=-100.250000, lat=-64.589706, depth=1.000000, time=0.000000)
P[48](lon=-100.150002, lat=-64.589706, depth=1.000000, time=0.000000)
P[49](lon=-100.050003, lat=-64.589706, depth=1.000000, time=0.000000)
P[50](lon=-100.449997, lat=-64.546768, depth=1.000000, time=0.000000)
P[51](lon=-100.349998, lat=-64.546768, depth=1.000000, time=0.000000)
P[52](lon=-100.250000, lat=-64.546768, depth=1.000000, time=0.000000)
P[53](lon=-100.150002, lat=-64.546768, depth=1.000000, time=0.000000)
P[54](lon=-100.050003, lat=-64.546768, depth=1.000000, time=0.000000)
P[55](lon=-100.449997, lat=-64.503754, depth=1.000000, time=0.000000)
P[56](lon=-100.349998, lat=-64.503754, depth=1.000000, time=0.000000)
P[57](lon=-100.250000, lat=-64.503754, depth=1.000000, time=0.000000)
P[58](lon=-100.150002, lat=-64.503754, depth=1.000000, time=0.000000)
P[59](lon=-100.050003, lat=-64.503754, depth=1.000000, time=0.000000)
P[60](lon=-100.449997, lat=-64.460678, depth=1.000000, time=0.000000)
P[61](lon=-100.349998, lat=-64.460678, depth=1.000000, time=0.000000)
P[62](lon=-100.250000, lat=-64.460678, depth=1.000000, time=0.000000)
P[63](lon=-100.150002, lat=-64.460678, depth=1.000000, time=0.000000)
P[64](lon=-100.050003, lat=-64.460678, depth=1.000000, time=0.000000)
P[65](lon=-100.449997, lat=-64.417526, depth=1.000000, time=0.000000)
P[66](lon=-100.349998, lat=-64.417526, depth=1.000000, time=0.000000)
P[67](lon=-100.250000, lat=-64.417526, depth=1.000000, time=0.000000)
P[68](lon=-100.150002, lat=-64.417526, depth=1.000000, time=0.000000)
P[69](lon=-100.050003, lat=-64.417526, depth=1.000000, time=0.000000)
P[70](lon=-100.449997, lat=-64.374313, depth=1.000000, time=0.000000)
P[71](lon=-100.349998, lat=-64.374313, depth=1.000000, time=0.000000)
P[72](lon=-100.250000, lat=-64.374313, depth=1.000000, time=0.000000)
P[73](lon=-100.150002, lat=-64.374313, depth=1.000000, time=0.000000)
P[74](lon=-100.050003, lat=-64.374313, depth=1.000000, time=0.000000)
P[75](lon=-100.449997, lat=-64.331032, depth=1.000000, time=0.000000)
P[76](lon=-100.349998, lat=-64.331032, depth=1.000000, time=0.000000)
P[77](lon=-100.250000, lat=-64.331032, depth=1.000000, time=0.000000)
P[78](lon=-100.150002, lat=-64.331032, depth=1.000000, time=0.000000)
P[79](lon=-100.050003, lat=-64.331032, depth=1.000000, time=0.000000)
P[80](lon=-100.449997, lat=-64.287682, depth=1.000000, time=0.000000)
P[81](lon=-100.349998, lat=-64.287682, depth=1.000000, time=0.000000)
P[82](lon=-100.250000, lat=-64.287682, depth=1.000000, time=0.000000)
P[83](lon=-100.150002, lat=-64.287682, depth=1.000000, time=0.000000)
P[84](lon=-100.050003, lat=-64.287682, depth=1.000000, time=0.000000)
P[85](lon=-100.449997, lat=-64.244263, depth=1.000000, time=0.000000)
P[86](lon=-100.349998, lat=-64.244263, depth=1.000000, time=0.000000)
P[87](lon=-100.250000, lat=-64.244263, depth=1.000000, time=0.000000)
P[88](lon=-100.150002, lat=-64.244263, depth=1.000000, time=0.000000)
P[89](lon=-100.050003, lat=-64.244263, depth=1.000000, time=0.000000)
P[90](lon=-100.449997, lat=-64.200775, depth=1.000000, time=0.000000)
P[91](lon=-100.349998, lat=-64.200775, depth=1.000000, time=0.000000)
P[92](lon=-100.250000, lat=-64.200775, depth=1.000000, time=0.000000)
P[93](lon=-100.150002, lat=-64.200775, depth=1.000000, time=0.000000)
P[94](lon=-100.050003, lat=-64.200775, depth=1.000000, time=0.000000)
P[95](lon=-100.449997, lat=-64.157219, depth=1.000000, time=0.000000)
P[96](lon=-100.349998, lat=-64.157219, depth=1.000000, time=0.000000)
P[97](lon=-100.250000, lat=-64.157219, depth=1.000000, time=0.000000)
P[98](lon=-100.150002, lat=-64.157219, depth=1.000000, time=0.000000)
P[99](lon=-100.050003, lat=-64.157219, depth=1.000000, time=0.000000)
P[100](lon=-100.449997, lat=-64.113594, depth=1.000000, time=0.000000)
P[101](lon=-100.349998, lat=-64.113594, depth=1.000000, time=0.000000)
P[102](lon=-100.250000, lat=-64.113594, depth=1.000000, time=0.000000)
P[103](lon=-100.150002, lat=-64.113594, depth=1.000000, time=0.000000)
P[104](lon=-100.050003, lat=-64.113594, depth=1.000000, time=0.000000)
P[105](lon=-100.449997, lat=-64.069901, depth=1.000000, time=0.000000)
P[106](lon=-100.349998, lat=-64.069901, depth=1.000000, time=0.000000)
P[107](lon=-100.250000, lat=-64.069901, depth=1.000000, time=0.000000)
P[108](lon=-100.150002, lat=-64.069901, depth=1.000000, time=0.000000)
P[109](lon=-100.050003, lat=-64.069901, depth=1.000000, time=0.000000)
P[110](lon=-100.449997, lat=-64.026138, depth=1.000000, time=0.000000)
P[111](lon=-100.349998, lat=-64.026138, depth=1.000000, time=0.000000)
P[112](lon=-100.250000, lat=-64.026138, depth=1.000000, time=0.000000)
P[113](lon=-100.150002, lat=-64.026138, depth=1.000000, time=0.000000)
P[114](lon=-100.050003, lat=-64.026138, depth=1.000000, time=0.000000)
P[115](lon=-100.449997, lat=-63.982307, depth=1.000000, time=0.000000)
P[116](lon=-100.349998, lat=-63.982307, depth=1.000000, time=0.000000)
P[117](lon=-100.250000, lat=-63.982307, depth=1.000000, time=0.000000)
P[118](lon=-100.150002, lat=-63.982307, depth=1.000000, time=0.000000)
P[119](lon=-100.050003, lat=-63.982307, depth=1.000000, time=0.000000)
P[120](lon=-100.449997, lat=-63.938408, depth=1.000000, time=0.000000)
P[121](lon=-100.349998, lat=-63.938408, depth=1.000000, time=0.000000)
P[122](lon=-100.250000, lat=-63.938408, depth=1.000000, time=0.000000)
P[123](lon=-100.150002, lat=-63.938408, depth=1.000000, time=0.000000)
P[124](lon=-100.050003, lat=-63.938408, depth=1.000000, time=0.000000)
P[125](lon=-100.449997, lat=-63.894440, depth=1.000000, time=0.000000)
P[126](lon=-100.349998, lat=-63.894440, depth=1.000000, time=0.000000)
P[127](lon=-100.250000, lat=-63.894440, depth=1.000000, time=0.000000)
P[128](lon=-100.150002, lat=-63.894440, depth=1.000000, time=0.000000)
P[129](lon=-100.050003, lat=-63.894440, depth=1.000000, time=0.000000)
P[130](lon=-100.449997, lat=-63.850403, depth=1.000000, time=0.000000)
P[131](lon=-100.349998, lat=-63.850403, depth=1.000000, time=0.000000)
P[132](lon=-100.250000, lat=-63.850403, depth=1.000000, time=0.000000)
P[133](lon=-100.150002, lat=-63.850403, depth=1.000000, time=0.000000)
P[134](lon=-100.050003, lat=-63.850403, depth=1.000000, time=0.000000)
P[135](lon=-100.449997, lat=-63.806293, depth=1.000000, time=0.000000)
P[136](lon=-100.349998, lat=-63.806293, depth=1.000000, time=0.000000)
P[137](lon=-100.250000, lat=-63.806293, depth=1.000000, time=0.000000)
P[138](lon=-100.150002, lat=-63.806293, depth=1.000000, time=0.000000)
P[139](lon=-100.050003, lat=-63.806293, depth=1.000000, time=0.000000)
P[140](lon=-100.449997, lat=-63.762119, depth=1.000000, time=0.000000)
P[141](lon=-100.349998, lat=-63.762119, depth=1.000000, time=0.000000)
P[142](lon=-100.250000, lat=-63.762119, depth=1.000000, time=0.000000)
P[143](lon=-100.150002, lat=-63.762119, depth=1.000000, time=0.000000)
P[144](lon=-100.050003, lat=-63.762119, depth=1.000000, time=0.000000)
P[145](lon=-100.449997, lat=-63.717876, depth=1.000000, time=0.000000)
P[146](lon=-100.349998, lat=-63.717876, depth=1.000000, time=0.000000)
P[147](lon=-100.250000, lat=-63.717876, depth=1.000000, time=0.000000)
P[148](lon=-100.150002, lat=-63.717876, depth=1.000000, time=0.000000)
P[149](lon=-100.050003, lat=-63.717876, depth=1.000000, time=0.000000)
P[150](lon=-100.449997, lat=-63.673561, depth=1.000000, time=0.000000)
P[151](lon=-100.349998, lat=-63.673561, depth=1.000000, time=0.000000)
P[152](lon=-100.250000, lat=-63.673561, depth=1.000000, time=0.000000)
P[153](lon=-100.150002, lat=-63.673561, depth=1.000000, time=0.000000)
P[154](lon=-100.050003, lat=-63.673561, depth=1.000000, time=0.000000)
P[155](lon=-100.449997, lat=-63.629177, depth=1.000000, time=0.000000)
P[156](lon=-100.349998, lat=-63.629177, depth=1.000000, time=0.000000)
P[157](lon=-100.250000, lat=-63.629177, depth=1.000000, time=0.000000)
P[158](lon=-100.150002, lat=-63.629177, depth=1.000000, time=0.000000)
P[159](lon=-100.050003, lat=-63.629177, depth=1.000000, time=0.000000)
P[160](lon=-100.449997, lat=-63.584724, depth=1.000000, time=0.000000)
P[161](lon=-100.349998, lat=-63.584724, depth=1.000000, time=0.000000)
P[162](lon=-100.250000, lat=-63.584724, depth=1.000000, time=0.000000)
P[163](lon=-100.150002, lat=-63.584724, depth=1.000000, time=0.000000)
P[164](lon=-100.050003, lat=-63.584724, depth=1.000000, time=0.000000)
P[165](lon=-100.449997, lat=-63.540203, depth=1.000000, time=0.000000)
P[166](lon=-100.349998, lat=-63.540203, depth=1.000000, time=0.000000)
P[167](lon=-100.250000, lat=-63.540203, depth=1.000000, time=0.000000)
P[168](lon=-100.150002, lat=-63.540203, depth=1.000000, time=0.000000)
P[169](lon=-100.050003, lat=-63.540203, depth=1.000000, time=0.000000)
P[170](lon=-100.449997, lat=-63.495613, depth=1.000000, time=0.000000)
P[171](lon=-100.349998, lat=-63.495613, depth=1.000000, time=0.000000)
P[172](lon=-100.250000, lat=-63.495613, depth=1.000000, time=0.000000)
P[173](lon=-100.150002, lat=-63.495613, depth=1.000000, time=0.000000)
P[174](lon=-100.050003, lat=-63.495613, depth=1.000000, time=0.000000)
P[175](lon=-100.449997, lat=-63.450951, depth=1.000000, time=0.000000)
P[176](lon=-100.349998, lat=-63.450951, depth=1.000000, time=0.000000)
P[177](lon=-100.250000, lat=-63.450951, depth=1.000000, time=0.000000)
P[178](lon=-100.150002, lat=-63.450951, depth=1.000000, time=0.000000)
P[179](lon=-100.050003, lat=-63.450951, depth=1.000000, time=0.000000)
P[180](lon=-100.449997, lat=-63.406219, depth=1.000000, time=0.000000)
P[181](lon=-100.349998, lat=-63.406219, depth=1.000000, time=0.000000)
P[182](lon=-100.250000, lat=-63.406219, depth=1.000000, time=0.000000)
P[183](lon=-100.150002, lat=-63.406219, depth=1.000000, time=0.000000)
P[184](lon=-100.050003, lat=-63.406219, depth=1.000000, time=0.000000)
P[185](lon=-100.449997, lat=-63.361420, depth=1.000000, time=0.000000)
P[186](lon=-100.349998, lat=-63.361420, depth=1.000000, time=0.000000)
P[187](lon=-100.250000, lat=-63.361420, depth=1.000000, time=0.000000)
P[188](lon=-100.150002, lat=-63.361420, depth=1.000000, time=0.000000)
P[189](lon=-100.050003, lat=-63.361420, depth=1.000000, time=0.000000)
P[190](lon=-100.449997, lat=-63.316547, depth=1.000000, time=0.000000)
P[191](lon=-100.349998, lat=-63.316547, depth=1.000000, time=0.000000)
P[192](lon=-100.250000, lat=-63.316547, depth=1.000000, time=0.000000)
P[193](lon=-100.150002, lat=-63.316547, depth=1.000000, time=0.000000)
P[194](lon=-100.050003, lat=-63.316547, depth=1.000000, time=0.000000)
P[195](lon=-100.449997, lat=-63.271606, depth=1.000000, time=0.000000)
P[196](lon=-100.349998, lat=-63.271606, depth=1.000000, time=0.000000)
P[197](lon=-100.250000, lat=-63.271606, depth=1.000000, time=0.000000)
P[198](lon=-100.150002, lat=-63.271606, depth=1.000000, time=0.000000)
P[199](lon=-100.050003, lat=-63.271606, depth=1.000000, time=0.000000)
P[200](lon=-100.449997, lat=-63.226593, depth=1.000000, time=0.000000)
P[201](lon=-100.349998, lat=-63.226593, depth=1.000000, time=0.000000)
P[202](lon=-100.250000, lat=-63.226593, depth=1.000000, time=0.000000)
P[203](lon=-100.150002, lat=-63.226593, depth=1.000000, time=0.000000)
P[204](lon=-100.050003, lat=-63.226593, depth=1.000000, time=0.000000)
P[205](lon=-100.449997, lat=-63.181515, depth=1.000000, time=0.000000)
P[206](lon=-100.349998, lat=-63.181515, depth=1.000000, time=0.000000)
P[207](lon=-100.250000, lat=-63.181515, depth=1.000000, time=0.000000)
P[208](lon=-100.150002, lat=-63.181515, depth=1.000000, time=0.000000)
P[209](lon=-100.050003, lat=-63.181515, depth=1.000000, time=0.000000)
P[210](lon=-100.449997, lat=-63.136360, depth=1.000000, time=0.000000)
P[211](lon=-100.349998, lat=-63.136360, depth=1.000000, time=0.000000)
P[212](lon=-100.250000, lat=-63.136360, depth=1.000000, time=0.000000)
P[213](lon=-100.150002, lat=-63.136360, depth=1.000000, time=0.000000)
P[214](lon=-100.050003, lat=-63.136360, depth=1.000000, time=0.000000)
P[215](lon=-100.449997, lat=-63.091141, depth=1.000000, time=0.000000)
P[216](lon=-100.349998, lat=-63.091141, depth=1.000000, time=0.000000)
P[217](lon=-100.250000, lat=-63.091141, depth=1.000000, time=0.000000)
P[218](lon=-100.150002, lat=-63.091141, depth=1.000000, time=0.000000)
P[219](lon=-100.050003, lat=-63.091141, depth=1.000000, time=0.000000)
P[220](lon=-100.449997, lat=-63.045845, depth=1.000000, time=0.000000)
P[221](lon=-100.349998, lat=-63.045845, depth=1.000000, time=0.000000)
P[222](lon=-100.250000, lat=-63.045845, depth=1.000000, time=0.000000)
P[223](lon=-100.150002, lat=-63.045845, depth=1.000000, time=0.000000)
P[224](lon=-100.050003, lat=-63.045845, depth=1.000000, time=0.000000)
P[225](lon=-100.449997, lat=-63.000484, depth=1.000000, time=0.000000)
P[226](lon=-100.349998, lat=-63.000484, depth=1.000000, time=0.000000)
P[227](lon=-100.250000, lat=-63.000484, depth=1.000000, time=0.000000)
P[228](lon=-100.150002, lat=-63.000484, depth=1.000000, time=0.000000)
P[229](lon=-100.050003, lat=-63.000484, depth=1.000000, time=0.000000)
P[230](lon=-100.449997, lat=-62.955051, depth=1.000000, time=0.000000)
P[231](lon=-100.349998, lat=-62.955051, depth=1.000000, time=0.000000)
P[232](lon=-100.250000, lat=-62.955051, depth=1.000000, time=0.000000)
P[233](lon=-100.150002, lat=-62.955051, depth=1.000000, time=0.000000)
P[234](lon=-100.050003, lat=-62.955051, depth=1.000000, time=0.000000)
P[235](lon=-100.449997, lat=-62.909546, depth=1.000000, time=0.000000)
P[236](lon=-100.349998, lat=-62.909546, depth=1.000000, time=0.000000)
P[237](lon=-100.250000, lat=-62.909546, depth=1.000000, time=0.000000)
P[238](lon=-100.150002, lat=-62.909546, depth=1.000000, time=0.000000)
P[239](lon=-100.050003, lat=-62.909546, depth=1.000000, time=0.000000)
P[240](lon=-100.449997, lat=-62.863972, depth=1.000000, time=0.000000)
P[241](lon=-100.349998, lat=-62.863972, depth=1.000000, time=0.000000)
P[242](lon=-100.250000, lat=-62.863972, depth=1.000000, time=0.000000)
P[243](lon=-100.150002, lat=-62.863972, depth=1.000000, time=0.000000)
P[244](lon=-100.050003, lat=-62.863972, depth=1.000000, time=0.000000)
P[245](lon=-100.449997, lat=-62.818325, depth=1.000000, time=0.000000)
P[246](lon=-100.349998, lat=-62.818325, depth=1.000000, time=0.000000)
P[247](lon=-100.250000, lat=-62.818325, depth=1.000000, time=0.000000)
P[248](lon=-100.150002, lat=-62.818325, depth=1.000000, time=0.000000)
P[249](lon=-100.050003, lat=-62.818325, depth=1.000000, time=0.000000)
P[250](lon=-100.449997, lat=-62.772610, depth=1.000000, time=0.000000)
P[251](lon=-100.349998, lat=-62.772610, depth=1.000000, time=0.000000)
P[252](lon=-100.250000, lat=-62.772610, depth=1.000000, time=0.000000)
P[253](lon=-100.150002, lat=-62.772610, depth=1.000000, time=0.000000)
P[254](lon=-100.050003, lat=-62.772610, depth=1.000000, time=0.000000)
P[255](lon=-100.449997, lat=-62.726822, depth=1.000000, time=0.000000)
P[256](lon=-100.349998, lat=-62.726822, depth=1.000000, time=0.000000)
P[257](lon=-100.250000, lat=-62.726822, depth=1.000000, time=0.000000)
P[258](lon=-100.150002, lat=-62.726822, depth=1.000000, time=0.000000)
P[259](lon=-100.050003, lat=-62.726822, depth=1.000000, time=0.000000)
P[260](lon=-100.449997, lat=-62.680962, depth=1.000000, time=0.000000)
P[261](lon=-100.349998, lat=-62.680962, depth=1.000000, time=0.000000)
P[262](lon=-100.250000, lat=-62.680962, depth=1.000000, time=0.000000)
P[263](lon=-100.150002, lat=-62.680962, depth=1.000000, time=0.000000)
P[264](lon=-100.050003, lat=-62.680962, depth=1.000000, time=0.000000)
P[265](lon=-100.449997, lat=-62.635033, depth=1.000000, time=0.000000)
P[266](lon=-100.349998, lat=-62.635033, depth=1.000000, time=0.000000)
P[267](lon=-100.250000, lat=-62.635033, depth=1.000000, time=0.000000)
P[268](lon=-100.150002, lat=-62.635033, depth=1.000000, time=0.000000)
P[269](lon=-100.050003, lat=-62.635033, depth=1.000000, time=0.000000)
P[270](lon=-100.449997, lat=-62.589031, depth=1.000000, time=0.000000)
P[271](lon=-100.349998, lat=-62.589031, depth=1.000000, time=0.000000)
P[272](lon=-100.250000, lat=-62.589031, depth=1.000000, time=0.000000)
P[273](lon=-100.150002, lat=-62.589031, depth=1.000000, time=0.000000)
P[274](lon=-100.050003, lat=-62.589031, depth=1.000000, time=0.000000)
P[275](lon=-100.449997, lat=-62.542957, depth=1.000000, time=0.000000)
P[276](lon=-100.349998, lat=-62.542957, depth=1.000000, time=0.000000)
P[277](lon=-100.250000, lat=-62.542957, depth=1.000000, time=0.000000)
P[278](lon=-100.150002, lat=-62.542957, depth=1.000000, time=0.000000)
P[279](lon=-100.050003, lat=-62.542957, depth=1.000000, time=0.000000)
P[280](lon=-100.449997, lat=-62.496815, depth=1.000000, time=0.000000)
P[281](lon=-100.349998, lat=-62.496815, depth=1.000000, time=0.000000)
P[282](lon=-100.250000, lat=-62.496815, depth=1.000000, time=0.000000)
P[283](lon=-100.150002, lat=-62.496815, depth=1.000000, time=0.000000)
P[284](lon=-100.050003, lat=-62.496815, depth=1.000000, time=0.000000)
P[285](lon=-100.449997, lat=-62.450600, depth=1.000000, time=0.000000)
P[286](lon=-100.349998, lat=-62.450600, depth=1.000000, time=0.000000)
P[287](lon=-100.250000, lat=-62.450600, depth=1.000000, time=0.000000)
P[288](lon=-100.150002, lat=-62.450600, depth=1.000000, time=0.000000)
P[289](lon=-100.050003, lat=-62.450600, depth=1.000000, time=0.000000)
P[290](lon=-100.449997, lat=-62.404312, depth=1.000000, time=0.000000)
P[291](lon=-100.349998, lat=-62.404312, depth=1.000000, time=0.000000)
P[292](lon=-100.250000, lat=-62.404312, depth=1.000000, time=0.000000)
P[293](lon=-100.150002, lat=-62.404312, depth=1.000000, time=0.000000)
P[294](lon=-100.050003, lat=-62.404312, depth=1.000000, time=0.000000)
P[295](lon=-100.449997, lat=-62.357952, depth=1.000000, time=0.000000)
P[296](lon=-100.349998, lat=-62.357952, depth=1.000000, time=0.000000)
P[297](lon=-100.250000, lat=-62.357952, depth=1.000000, time=0.000000)
P[298](lon=-100.150002, lat=-62.357952, depth=1.000000, time=0.000000)
P[299](lon=-100.050003, lat=-62.357952, depth=1.000000, time=0.000000)
P[300](lon=-100.449997, lat=-62.311520, depth=1.000000, time=0.000000)
P[301](lon=-100.349998, lat=-62.311520, depth=1.000000, time=0.000000)
P[302](lon=-100.250000, lat=-62.311520, depth=1.000000, time=0.000000)
P[303](lon=-100.150002, lat=-62.311520, depth=1.000000, time=0.000000)
P[304](lon=-100.050003, lat=-62.311520, depth=1.000000, time=0.000000)
P[305](lon=-100.449997, lat=-62.265018, depth=1.000000, time=0.000000)
P[306](lon=-100.349998, lat=-62.265018, depth=1.000000, time=0.000000)
P[307](lon=-100.250000, lat=-62.265018, depth=1.000000, time=0.000000)
P[308](lon=-100.150002, lat=-62.265018, depth=1.000000, time=0.000000)
P[309](lon=-100.050003, lat=-62.265018, depth=1.000000, time=0.000000)
P[310](lon=-100.449997, lat=-62.218445, depth=1.000000, time=0.000000)
P[311](lon=-100.349998, lat=-62.218445, depth=1.000000, time=0.000000)
P[312](lon=-100.250000, lat=-62.218445, depth=1.000000, time=0.000000)
P[313](lon=-100.150002, lat=-62.218445, depth=1.000000, time=0.000000)
P[314](lon=-100.050003, lat=-62.218445, depth=1.000000, time=0.000000)
P[315](lon=-100.449997, lat=-62.171799, depth=1.000000, time=0.000000)
P[316](lon=-100.349998, lat=-62.171799, depth=1.000000, time=0.000000)
P[317](lon=-100.250000, lat=-62.171799, depth=1.000000, time=0.000000)
P[318](lon=-100.150002, lat=-62.171799, depth=1.000000, time=0.000000)
P[319](lon=-100.050003, lat=-62.171799, depth=1.000000, time=0.000000)
P[320](lon=-100.449997, lat=-62.125080, depth=1.000000, time=0.000000)
P[321](lon=-100.349998, lat=-62.125080, depth=1.000000, time=0.000000)
P[322](lon=-100.250000, lat=-62.125080, depth=1.000000, time=0.000000)
P[323](lon=-100.150002, lat=-62.125080, depth=1.000000, time=0.000000)
P[324](lon=-100.050003, lat=-62.125080, depth=1.000000, time=0.000000)
P[325](lon=-100.449997, lat=-62.078289, depth=1.000000, time=0.000000)
P[326](lon=-100.349998, lat=-62.078289, depth=1.000000, time=0.000000)
P[327](lon=-100.250000, lat=-62.078289, depth=1.000000, time=0.000000)
P[328](lon=-100.150002, lat=-62.078289, depth=1.000000, time=0.000000)
P[329](lon=-100.050003, lat=-62.078289, depth=1.000000, time=0.000000)
P[330](lon=-100.449997, lat=-62.031429, depth=1.000000, time=0.000000)
P[331](lon=-100.349998, lat=-62.031429, depth=1.000000, time=0.000000)
P[332](lon=-100.250000, lat=-62.031429, depth=1.000000, time=0.000000)
P[333](lon=-100.150002, lat=-62.031429, depth=1.000000, time=0.000000)
P[334](lon=-100.050003, lat=-62.031429, depth=1.000000, time=0.000000)
P[335](lon=-100.449997, lat=-61.984493, depth=1.000000, time=0.000000)
P[336](lon=-100.349998, lat=-61.984493, depth=1.000000, time=0.000000)
P[337](lon=-100.250000, lat=-61.984493, depth=1.000000, time=0.000000)
P[338](lon=-100.150002, lat=-61.984493, depth=1.000000, time=0.000000)
P[339](lon=-100.050003, lat=-61.984493, depth=1.000000, time=0.000000)
P[340](lon=-100.449997, lat=-61.937485, depth=1.000000, time=0.000000)
P[341](lon=-100.349998, lat=-61.937485, depth=1.000000, time=0.000000)
P[342](lon=-100.250000, lat=-61.937485, depth=1.000000, time=0.000000)
P[343](lon=-100.150002, lat=-61.937485, depth=1.000000, time=0.000000)
P[344](lon=-100.050003, lat=-61.937485, depth=1.000000, time=0.000000)
P[345](lon=-100.449997, lat=-61.890408, depth=1.000000, time=0.000000)
P[346](lon=-100.349998, lat=-61.890408, depth=1.000000, time=0.000000)
P[347](lon=-100.250000, lat=-61.890408, depth=1.000000, time=0.000000)
P[348](lon=-100.150002, lat=-61.890408, depth=1.000000, time=0.000000)
P[349](lon=-100.050003, lat=-61.890408, depth=1.000000, time=0.000000)
P[350](lon=-100.449997, lat=-61.843254, depth=1.000000, time=0.000000)
P[351](lon=-100.349998, lat=-61.843254, depth=1.000000, time=0.000000)
P[352](lon=-100.250000, lat=-61.843254, depth=1.000000, time=0.000000)
P[353](lon=-100.150002, lat=-61.843254, depth=1.000000, time=0.000000)
P[354](lon=-100.050003, lat=-61.843254, depth=1.000000, time=0.000000)
P[355](lon=-100.449997, lat=-61.796028, depth=1.000000, time=0.000000)
P[356](lon=-100.349998, lat=-61.796028, depth=1.000000, time=0.000000)
P[357](lon=-100.250000, lat=-61.796028, depth=1.000000, time=0.000000)
P[358](lon=-100.150002, lat=-61.796028, depth=1.000000, time=0.000000)
P[359](lon=-100.050003, lat=-61.796028, depth=1.000000, time=0.000000)
P[360](lon=-100.449997, lat=-61.748730, depth=1.000000, time=0.000000)
P[361](lon=-100.349998, lat=-61.748730, depth=1.000000, time=0.000000)
P[362](lon=-100.250000, lat=-61.748730, depth=1.000000, time=0.000000)
P[363](lon=-100.150002, lat=-61.748730, depth=1.000000, time=0.000000)
P[364](lon=-100.050003, lat=-61.748730, depth=1.000000, time=0.000000)
P[365](lon=-100.449997, lat=-61.701363, depth=1.000000, time=0.000000)
P[366](lon=-100.349998, lat=-61.701363, depth=1.000000, time=0.000000)
P[367](lon=-100.250000, lat=-61.701363, depth=1.000000, time=0.000000)
P[368](lon=-100.150002, lat=-61.701363, depth=1.000000, time=0.000000)
P[369](lon=-100.050003, lat=-61.701363, depth=1.000000, time=0.000000)
P[370](lon=-100.449997, lat=-61.653919, depth=1.000000, time=0.000000)
P[371](lon=-100.349998, lat=-61.653919, depth=1.000000, time=0.000000)
P[372](lon=-100.250000, lat=-61.653919, depth=1.000000, time=0.000000)
P[373](lon=-100.150002, lat=-61.653919, depth=1.000000, time=0.000000)
P[374](lon=-100.050003, lat=-61.653919, depth=1.000000, time=0.000000)
P[375](lon=-100.449997, lat=-61.606403, depth=1.000000, time=0.000000)
P[376](lon=-100.349998, lat=-61.606403, depth=1.000000, time=0.000000)
P[377](lon=-100.250000, lat=-61.606403, depth=1.000000, time=0.000000)
P[378](lon=-100.150002, lat=-61.606403, depth=1.000000, time=0.000000)
P[379](lon=-100.050003, lat=-61.606403, depth=1.000000, time=0.000000)
P[380](lon=-100.449997, lat=-61.558811, depth=1.000000, time=0.000000)
P[381](lon=-100.349998, lat=-61.558811, depth=1.000000, time=0.000000)
P[382](lon=-100.250000, lat=-61.558811, depth=1.000000, time=0.000000)
P[383](lon=-100.150002, lat=-61.558811, depth=1.000000, time=0.000000)
P[384](lon=-100.050003, lat=-61.558811, depth=1.000000, time=0.000000)
P[385](lon=-100.449997, lat=-61.511150, depth=1.000000, time=0.000000)
P[386](lon=-100.349998, lat=-61.511150, depth=1.000000, time=0.000000)
P[387](lon=-100.250000, lat=-61.511150, depth=1.000000, time=0.000000)
P[388](lon=-100.150002, lat=-61.511150, depth=1.000000, time=0.000000)
P[389](lon=-100.050003, lat=-61.511150, depth=1.000000, time=0.000000)
P[390](lon=-100.449997, lat=-61.463413, depth=1.000000, time=0.000000)
P[391](lon=-100.349998, lat=-61.463413, depth=1.000000, time=0.000000)
P[392](lon=-100.250000, lat=-61.463413, depth=1.000000, time=0.000000)
P[393](lon=-100.150002, lat=-61.463413, depth=1.000000, time=0.000000)
P[394](lon=-100.050003, lat=-61.463413, depth=1.000000, time=0.000000)
P[395](lon=-100.449997, lat=-61.415607, depth=1.000000, time=0.000000)
P[396](lon=-100.349998, lat=-61.415607, depth=1.000000, time=0.000000)
P[397](lon=-100.250000, lat=-61.415607, depth=1.000000, time=0.000000)
P[398](lon=-100.150002, lat=-61.415607, depth=1.000000, time=0.000000)
P[399](lon=-100.050003, lat=-61.415607, depth=1.000000, time=0.000000)
P[400](lon=-100.449997, lat=-61.367725, depth=1.000000, time=0.000000)
P[401](lon=-100.349998, lat=-61.367725, depth=1.000000, time=0.000000)
P[402](lon=-100.250000, lat=-61.367725, depth=1.000000, time=0.000000)
P[403](lon=-100.150002, lat=-61.367725, depth=1.000000, time=0.000000)
P[404](lon=-100.050003, lat=-61.367725, depth=1.000000, time=0.000000)
P[405](lon=-100.449997, lat=-61.319771, depth=1.000000, time=0.000000)
P[406](lon=-100.349998, lat=-61.319771, depth=1.000000, time=0.000000)
P[407](lon=-100.250000, lat=-61.319771, depth=1.000000, time=0.000000)
P[408](lon=-100.150002, lat=-61.319771, depth=1.000000, time=0.000000)
P[409](lon=-100.050003, lat=-61.319771, depth=1.000000, time=0.000000)
P[410](lon=-100.449997, lat=-61.271740, depth=1.000000, time=0.000000)
P[411](lon=-100.349998, lat=-61.271740, depth=1.000000, time=0.000000)
P[412](lon=-100.250000, lat=-61.271740, depth=1.000000, time=0.000000)
P[413](lon=-100.150002, lat=-61.271740, depth=1.000000, time=0.000000)
P[414](lon=-100.050003, lat=-61.271740, depth=1.000000, time=0.000000)
P[415](lon=-100.449997, lat=-61.223637, depth=1.000000, time=0.000000)
P[416](lon=-100.349998, lat=-61.223637, depth=1.000000, time=0.000000)
P[417](lon=-100.250000, lat=-61.223637, depth=1.000000, time=0.000000)
P[418](lon=-100.150002, lat=-61.223637, depth=1.000000, time=0.000000)
P[419](lon=-100.050003, lat=-61.223637, depth=1.000000, time=0.000000)
P[420](lon=-100.449997, lat=-61.175461, depth=1.000000, time=0.000000)
P[421](lon=-100.349998, lat=-61.175461, depth=1.000000, time=0.000000)
P[422](lon=-100.250000, lat=-61.175461, depth=1.000000, time=0.000000)
P[423](lon=-100.150002, lat=-61.175461, depth=1.000000, time=0.000000)
P[424](lon=-100.050003, lat=-61.175461, depth=1.000000, time=0.000000)
P[425](lon=-100.449997, lat=-61.127213, depth=1.000000, time=0.000000)
P[426](lon=-100.349998, lat=-61.127213, depth=1.000000, time=0.000000)
P[427](lon=-100.250000, lat=-61.127213, depth=1.000000, time=0.000000)
P[428](lon=-100.150002, lat=-61.127213, depth=1.000000, time=0.000000)
P[429](lon=-100.050003, lat=-61.127213, depth=1.000000, time=0.000000)
P[430](lon=-100.449997, lat=-61.078888, depth=1.000000, time=0.000000)
P[431](lon=-100.349998, lat=-61.078888, depth=1.000000, time=0.000000)
P[432](lon=-100.250000, lat=-61.078888, depth=1.000000, time=0.000000)
P[433](lon=-100.150002, lat=-61.078888, depth=1.000000, time=0.000000)
P[434](lon=-100.050003, lat=-61.078888, depth=1.000000, time=0.000000)
P[435](lon=-100.449997, lat=-61.030491, depth=1.000000, time=0.000000)
P[436](lon=-100.349998, lat=-61.030491, depth=1.000000, time=0.000000)
P[437](lon=-100.250000, lat=-61.030491, depth=1.000000, time=0.000000)
P[438](lon=-100.150002, lat=-61.030491, depth=1.000000, time=0.000000)
P[439](lon=-100.050003, lat=-61.030491, depth=1.000000, time=0.000000)
P[440](lon=-100.449997, lat=-60.982021, depth=1.000000, time=0.000000)
P[441](lon=-100.349998, lat=-60.982021, depth=1.000000, time=0.000000)
P[442](lon=-100.250000, lat=-60.982021, depth=1.000000, time=0.000000)
P[443](lon=-100.150002, lat=-60.982021, depth=1.000000, time=0.000000)
P[444](lon=-100.050003, lat=-60.982021, depth=1.000000, time=0.000000)
P[445](lon=-100.449997, lat=-60.933475, depth=1.000000, time=0.000000)
P[446](lon=-100.349998, lat=-60.933475, depth=1.000000, time=0.000000)
P[447](lon=-100.250000, lat=-60.933475, depth=1.000000, time=0.000000)
P[448](lon=-100.150002, lat=-60.933475, depth=1.000000, time=0.000000)
P[449](lon=-100.050003, lat=-60.933475, depth=1.000000, time=0.000000)
P[450](lon=-100.449997, lat=-60.884853, depth=1.000000, time=0.000000)
P[451](lon=-100.349998, lat=-60.884853, depth=1.000000, time=0.000000)
P[452](lon=-100.250000, lat=-60.884853, depth=1.000000, time=0.000000)
P[453](lon=-100.150002, lat=-60.884853, depth=1.000000, time=0.000000)
P[454](lon=-100.050003, lat=-60.884853, depth=1.000000, time=0.000000)
P[455](lon=-100.449997, lat=-60.836163, depth=1.000000, time=0.000000)
P[456](lon=-100.349998, lat=-60.836163, depth=1.000000, time=0.000000)
P[457](lon=-100.250000, lat=-60.836163, depth=1.000000, time=0.000000)
P[458](lon=-100.150002, lat=-60.836163, depth=1.000000, time=0.000000)
P[459](lon=-100.050003, lat=-60.836163, depth=1.000000, time=0.000000)
P[460](lon=-100.449997, lat=-60.787392, depth=1.000000, time=0.000000)
P[461](lon=-100.349998, lat=-60.787392, depth=1.000000, time=0.000000)
P[462](lon=-100.250000, lat=-60.787392, depth=1.000000, time=0.000000)
P[463](lon=-100.150002, lat=-60.787392, depth=1.000000, time=0.000000)
P[464](lon=-100.050003, lat=-60.787392, depth=1.000000, time=0.000000)
P[465](lon=-100.449997, lat=-60.738552, depth=1.000000, time=0.000000)
P[466](lon=-100.349998, lat=-60.738552, depth=1.000000, time=0.000000)
P[467](lon=-100.250000, lat=-60.738552, depth=1.000000, time=0.000000)
P[468](lon=-100.150002, lat=-60.738552, depth=1.000000, time=0.000000)
P[469](lon=-100.050003, lat=-60.738552, depth=1.000000, time=0.000000)
P[470](lon=-100.449997, lat=-60.689632, depth=1.000000, time=0.000000)
P[471](lon=-100.349998, lat=-60.689632, depth=1.000000, time=0.000000)
P[472](lon=-100.250000, lat=-60.689632, depth=1.000000, time=0.000000)
P[473](lon=-100.150002, lat=-60.689632, depth=1.000000, time=0.000000)
P[474](lon=-100.050003, lat=-60.689632, depth=1.000000, time=0.000000)
P[475](lon=-100.449997, lat=-60.640644, depth=1.000000, time=0.000000)
P[476](lon=-100.349998, lat=-60.640644, depth=1.000000, time=0.000000)
P[477](lon=-100.250000, lat=-60.640644, depth=1.000000, time=0.000000)
P[478](lon=-100.150002, lat=-60.640644, depth=1.000000, time=0.000000)
P[479](lon=-100.050003, lat=-60.640644, depth=1.000000, time=0.000000)
P[480](lon=-100.449997, lat=-60.591576, depth=1.000000, time=0.000000)
P[481](lon=-100.349998, lat=-60.591576, depth=1.000000, time=0.000000)
P[482](lon=-100.250000, lat=-60.591576, depth=1.000000, time=0.000000)
P[483](lon=-100.150002, lat=-60.591576, depth=1.000000, time=0.000000)
P[484](lon=-100.050003, lat=-60.591576, depth=1.000000, time=0.000000)
P[485](lon=-100.449997, lat=-60.542435, depth=1.000000, time=0.000000)
P[486](lon=-100.349998, lat=-60.542435, depth=1.000000, time=0.000000)
P[487](lon=-100.250000, lat=-60.542435, depth=1.000000, time=0.000000)
P[488](lon=-100.150002, lat=-60.542435, depth=1.000000, time=0.000000)
P[489](lon=-100.050003, lat=-60.542435, depth=1.000000, time=0.000000)
P[490](lon=-100.449997, lat=-60.493221, depth=1.000000, time=0.000000)
P[491](lon=-100.349998, lat=-60.493221, depth=1.000000, time=0.000000)
P[492](lon=-100.250000, lat=-60.493221, depth=1.000000, time=0.000000)
P[493](lon=-100.150002, lat=-60.493221, depth=1.000000, time=0.000000)
P[494](lon=-100.050003, lat=-60.493221, depth=1.000000, time=0.000000)
P[495](lon=-100.449997, lat=-60.443932, depth=1.000000, time=0.000000)
P[496](lon=-100.349998, lat=-60.443932, depth=1.000000, time=0.000000)
P[497](lon=-100.250000, lat=-60.443932, depth=1.000000, time=0.000000)
P[498](lon=-100.150002, lat=-60.443932, depth=1.000000, time=0.000000)
P[499](lon=-100.050003, lat=-60.443932, depth=1.000000, time=0.000000)
P[500](lon=-100.449997, lat=-60.394566, depth=1.000000, time=0.000000)
P[501](lon=-100.349998, lat=-60.394566, depth=1.000000, time=0.000000)
P[502](lon=-100.250000, lat=-60.394566, depth=1.000000, time=0.000000)
P[503](lon=-100.150002, lat=-60.394566, depth=1.000000, time=0.000000)
P[504](lon=-100.050003, lat=-60.394566, depth=1.000000, time=0.000000)
P[505](lon=-100.449997, lat=-60.345127, depth=1.000000, time=0.000000)
P[506](lon=-100.349998, lat=-60.345127, depth=1.000000, time=0.000000)
P[507](lon=-100.250000, lat=-60.345127, depth=1.000000, time=0.000000)
P[508](lon=-100.150002, lat=-60.345127, depth=1.000000, time=0.000000)
P[509](lon=-100.050003, lat=-60.345127, depth=1.000000, time=0.000000)
P[510](lon=-100.449997, lat=-60.295612, depth=1.000000, time=0.000000)
P[511](lon=-100.349998, lat=-60.295612, depth=1.000000, time=0.000000)
P[512](lon=-100.250000, lat=-60.295612, depth=1.000000, time=0.000000)
P[513](lon=-100.150002, lat=-60.295612, depth=1.000000, time=0.000000)
P[514](lon=-100.050003, lat=-60.295612, depth=1.000000, time=0.000000)
P[515](lon=-100.449997, lat=-60.246021, depth=1.000000, time=0.000000)
P[516](lon=-100.349998, lat=-60.246021, depth=1.000000, time=0.000000)
P[517](lon=-100.250000, lat=-60.246021, depth=1.000000, time=0.000000)
P[518](lon=-100.150002, lat=-60.246021, depth=1.000000, time=0.000000)
P[519](lon=-100.050003, lat=-60.246021, depth=1.000000, time=0.000000)
P[520](lon=-100.449997, lat=-60.196358, depth=1.000000, time=0.000000)
P[521](lon=-100.349998, lat=-60.196358, depth=1.000000, time=0.000000)
P[522](lon=-100.250000, lat=-60.196358, depth=1.000000, time=0.000000)
P[523](lon=-100.150002, lat=-60.196358, depth=1.000000, time=0.000000)
P[524](lon=-100.050003, lat=-60.196358, depth=1.000000, time=0.000000)
P[525](lon=-100.449997, lat=-60.146614, depth=1.000000, time=0.000000)
P[526](lon=-100.349998, lat=-60.146614, depth=1.000000, time=0.000000)
P[527](lon=-100.250000, lat=-60.146614, depth=1.000000, time=0.000000)
P[528](lon=-100.150002, lat=-60.146614, depth=1.000000, time=0.000000)
P[529](lon=-100.050003, lat=-60.146614, depth=1.000000, time=0.000000)
P[530](lon=-100.449997, lat=-60.096798, depth=1.000000, time=0.000000)
P[531](lon=-100.349998, lat=-60.096798, depth=1.000000, time=0.000000)
P[532](lon=-100.250000, lat=-60.096798, depth=1.000000, time=0.000000)
P[533](lon=-100.150002, lat=-60.096798, depth=1.000000, time=0.000000)
P[534](lon=-100.050003, lat=-60.096798, depth=1.000000, time=0.000000)
P[535](lon=-100.449997, lat=-60.046909, depth=1.000000, time=0.000000)
P[536](lon=-100.349998, lat=-60.046909, depth=1.000000, time=0.000000)
P[537](lon=-100.250000, lat=-60.046909, depth=1.000000, time=0.000000)
P[538](lon=-100.150002, lat=-60.046909, depth=1.000000, time=0.000000)
P[539](lon=-100.050003, lat=-60.046909, depth=1.000000, time=0.000000)
Plot these particles.
[12]:
fig = plt.figure(figsize=(12, 4))
ht.plot(cmap='Blues')
plt.scatter(lons, lats, s=5, c='r')
plt.ylim([-80, -35]);
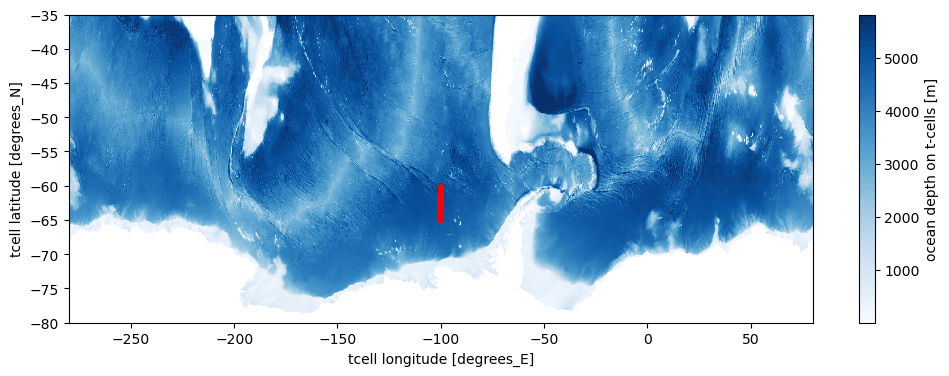
We can now advect these particles with Parcels. We’ll do this using 4th order Runge-Kutta in 2D. In this example, we’ll integrate the particle positions for only 50 days to keep it simple. This may take a few minutes depending on the resources you’re using. Feel free to change the total length of the run as you like.
Note: This cell might take 3-4 minutes.
[13]:
# Set your output location:
output_filename = 'TestParticles_2D.zarr'
# the file name and the time step of the outputs
# (particle locations will be saved every 5 days in this example)
output_file = pset.ParticleFile(
name=output_directory + output_filename,
outputdt=timedelta(days=2)
)
pset.execute(
[
AdvectionRK4, # the 2D advection kernel (which defines how particles move) at least one of these needs the pset.Kernel() surrounding it.
periodicBC ,
checkOutOfBounds
],
runtime=timedelta(days=50), # the total length of the run
dt=timedelta(hours=2), # the integration timestep of the kernels
output_file=output_file, # the output file
)
INFO: Output files are stored in /scratch/tm70/as2285/particle_trackingTestParticles_2D.zarr.
100%|██████████| 4320000.0/4320000.0 [01:14<00:00, 58373.79it/s]
Open output file and plot the first 100 of these trajectories.
[14]:
ds = xr.open_zarr(output_directory + output_filename)
fig = plt.figure(figsize=(12, 8))
mpl.rcParams['axes.prop_cycle'] = mpl.cycler(color=["r", "pink", "violet", "firebrick", "orange", "orangered"])
ht.plot(cmap='Blues')
plt.plot(ds.lon[:100, :].T, ds.lat[:100, :].T)
plt.scatter(ds.lon[:100, :], ds.lat[:100, :], s=5, c='w')
plt.ylim([-68, -60])
plt.xlim([-108, -90]);
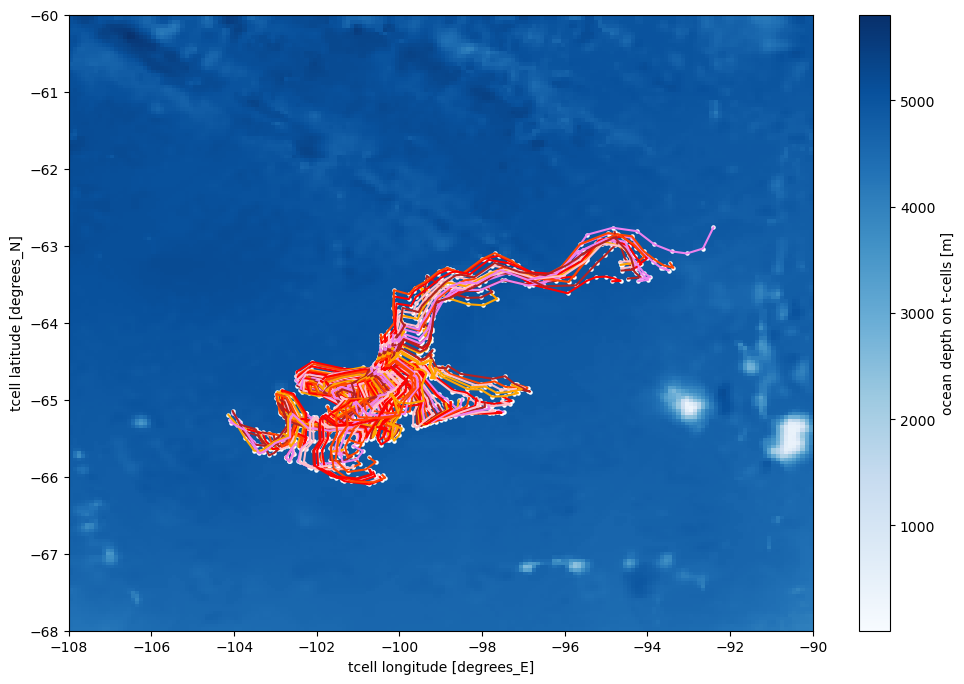
3D Advection with temperature and salinity¶
Now we’ll run an example in 3D and also sample and save temperature and salinity fields.
[15]:
# First, we need to define ACCESS-OM2-01 (MOM5 ocean) NetCDF files.
data_path = '/g/data/ik11/outputs/access-om2-01/01deg_jra55v13_ryf9091/'
# At a minimum, Parcels needs U and V files (2D advection), and W for 3D advection.
ufiles = sorted(glob(data_path+'output19*/ocean/ocean_daily_3d_u_*.nc'))
vfiles = sorted(glob(data_path+'output19*/ocean/ocean_daily_3d_v_*.nc'))
wfiles = sorted(glob(data_path+'output19*/ocean/ocean_daily_3d_wt_*.nc'))
# Optional
# You can also feed Parcels temperature, salinity, and 2D fields such as MLD.
# These should be at the same temporal resolution as the U, V and W fields.
tfiles = sorted(glob(data_path+'output19*/ocean/ocean_daily_3d_temp_*.nc'))
sfiles = sorted(glob(data_path+'output19*/ocean/ocean_daily_3d_salt_*.nc'))
# Read in the grid coordinates
# NOTE: If you haven't already created this file in the 2D advection example, then you need to
# do so before moving on.
mesh_mask = output_directory+'ocean.nc'
We now need to set up the Parcels FieldSet
. For 3D advection we also need a vertical velocity field (W
), and in this example we’ll add temperature and salinity as well.
Note: Initialising the FieldSet
can take some time depending on the domain and number of files you’re feeding to Parcels. In this example, it should be quick.
[16]:
%%time
# We now define a dictionary that tells Parcels where the U,V data is, and where to
# find the coordinates.
filenames = {'U': {'lon': mesh_mask, 'lat': mesh_mask, 'depth': mesh_mask, 'data': ufiles},
'V': {'lon': mesh_mask, 'lat': mesh_mask, 'depth': mesh_mask, 'data': vfiles},
'W': {'lon': mesh_mask, 'lat': mesh_mask, 'depth': mesh_mask, 'data': wfiles},
'T': {'lon': mesh_mask, 'lat': mesh_mask, 'depth': mesh_mask, 'data': tfiles},
'S': {'lon': mesh_mask, 'lat': mesh_mask, 'depth': mesh_mask, 'data': sfiles},
}
# Now define a dictionary that specifies the (`U,V,W` etc) variable names as given in the netcdf files
variables = {'U': 'u',
'V': 'v',
'W': 'wt',
'T': 'temp',
'S': 'salt',
}
# Define a dictionary to specify the U,V, W etc dimentions.
# All variables must have the same lat/lon/depth dimensions (even though the ACCESS-OM2-01 data doesn't).
# When we developed the from_mom5() method, Parcels expected the dimensions on the
# upper-right verticies of the grid cells. Hoewever, in ACCESS-OM2-01, the vertical
# velocity is defined on the bottom face of the grid cell, not the top. Therefore
# the from_mom5() method adds a layer to the top of the vertical velocity field such that wt = 0 @ 0m,
# so that the vertical velocities are correctly defined at the top surface of the grid cells.
dimensions = {'U': {'lon': 'xu_ocean', 'lat': 'yu_ocean', 'depth': 'sw_ocean', 'time': 'time'},
'V': {'lon': 'xu_ocean', 'lat': 'yu_ocean', 'depth': 'sw_ocean', 'time': 'time'},
'W': {'lon': 'xu_ocean', 'lat': 'yu_ocean', 'depth': 'sw_ocean', 'time': 'time'},
'T': {'lon': 'xu_ocean', 'lat': 'yu_ocean', 'depth': 'sw_ocean', 'time': 'time'},
'S': {'lon': 'xu_ocean', 'lat': 'yu_ocean', 'depth': 'sw_ocean', 'time': 'time'},
}
# Define the chunksizes for each variable. This will help Parcels read in the FieldSet.
cs = {"U": {"lon": ("xu_ocean", 400), "lat": ("yu_ocean", 300), "depth": ("st_ocean", 7), "time": ("time", 1)},
"V": {"lon": ("xu_ocean", 400), "lat": ("yu_ocean", 300), "depth": ("st_ocean", 7), "time": ("time", 1)},
"W": {"lon": ("xt_ocean", 400), "lat": ("yt_ocean", 300), "depth": ("sw_ocean", 7), "time": ("time", 1)},
"T": {"lon": ("xt_ocean", 400), "lat": ("yt_ocean", 300), "depth": ("st_ocean", 7), "time": ("time", 1)},
"S": {"lon": ("xt_ocean", 400), "lat": ("yt_ocean", 300), "depth": ("st_ocean", 7), "time": ("time", 1)},
}
# Finally, we load the fieldset using the Parcels `FieldSet.from_mom5` function.
fieldset = FieldSet.from_mom5(filenames, variables, dimensions, #indices,
mesh = 'spherical',
chunksize=cs,
tracer_interp_method = 'bgrid_tracer')
# Finally, we load the fieldset using the Parcels `FieldSet.from_mom5` function. This may take some time.
# This was adapted from the `from_b_grid_dataset` method to work with ACCESS-OM2-01.
# Note that W is normally directed upward in MOM5 (the ocean component of ACCESS-OM2-01),
# but Parcels requires W to be aligned with the positive z-direction (downward in MOM5). As such,
# the W fields are multiplied by -1 in this method.
fieldset = FieldSet.from_mom5(filenames, variables, dimensions,
mesh = 'spherical',
chunksize = cs,
tracer_interp_method = 'bgrid_tracer')
CPU times: user 2.21 s, sys: 82.8 ms, total: 2.29 s
Wall time: 2.28 s
As for the 2D advection case, we need to tell Parcels that this fieldset is periodic in the zonal (east-west) direction. This is because, if the particle is close to the edge of the fieldset (but still in it), the advection scheme will need to interpolate velocities that may lay outside the fieldset domain. With the halo, we make sure the advection kernel can access these values.
[17]:
fieldset.add_constant('halo_west', fieldset.U.grid.lon[0])
fieldset.add_constant('halo_east', fieldset.U.grid.lon[-1])
fieldset.add_periodic_halo(zonal=True)
We now need to define a particle class that tells Parcels each particle has a temperature and salinity value associated with it. We also need to define a kernel to tell Parcels to sample the temperature and salinity fields at each integration timestep.
[18]:
# Parcels will automatically save the latitude, longitude, and depth to file at a timestep that
# you specify (see cells below). This kernel tells Parcels to also save the temperature and salinity fields
# to file, along with the location data.
class SampleParticle(JITParticle):
thermo = Variable('thermo', dtype=np.float32, initial = 0.)
psal = Variable('psal', dtype=np.float32, initial = 0.)
# Kernel to sample temperature and salinity (if you have fed them into your FieldSet above).
def SampleFields(particle, fieldset, time):
particle.thermo = fieldset.T[time, particle.depth, particle.lat, particle.lon]
particle.psal = fieldset.S[time, particle.depth, particle.lat, particle.lon]
Now we can create the Particle Set. Well use the same particle set as in the 2D example but this time we’ll initialise particles at 10m depth. This time you can see that thermo
and psal
have been added to the Particle Set.
[19]:
session = cc.database.create_session()
experiment = '01deg_jra55v13_ryf9091'
ht = cc.querying.getvar(experiment, 'ht', session, n=1).load()
x1, x2 = -100.5, -100
y1, y2 = -60, -65
lons = ht.where((ht.yt_ocean<y1)&(ht.yt_ocean>y2)&(ht.xt_ocean<x2)&(ht.xt_ocean>x1),
drop=True).geolon_t.values.ravel()
lats = ht.where((ht.yt_ocean<y1)&(ht.yt_ocean>y2)&(ht.xt_ocean<x2)&(ht.xt_ocean>x1),
drop=True).geolat_t.values.ravel()
depths = np.full(len(lats), 10.)
pset = ParticleSet.from_list(fieldset=fieldset, # the fields on which the particles are advected
pclass=SampleParticle, # the type of particles we specified above with temp and salt added
lon=lons, # a vector of release longitudes
lat=lats, # a vector of release latitudes
depth=depths, # a vector of release depths
time=0.) # set time to start start time of daily velocity fields
pset
[19]:
P[540](lon=-100.449997, lat=-64.973167, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[541](lon=-100.349998, lat=-64.973167, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[542](lon=-100.250000, lat=-64.973167, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[543](lon=-100.150002, lat=-64.973167, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[544](lon=-100.050003, lat=-64.973167, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[545](lon=-100.449997, lat=-64.930832, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[546](lon=-100.349998, lat=-64.930832, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[547](lon=-100.250000, lat=-64.930832, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[548](lon=-100.150002, lat=-64.930832, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[549](lon=-100.050003, lat=-64.930832, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[550](lon=-100.449997, lat=-64.888428, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[551](lon=-100.349998, lat=-64.888428, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[552](lon=-100.250000, lat=-64.888428, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[553](lon=-100.150002, lat=-64.888428, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[554](lon=-100.050003, lat=-64.888428, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[555](lon=-100.449997, lat=-64.845955, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[556](lon=-100.349998, lat=-64.845955, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[557](lon=-100.250000, lat=-64.845955, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[558](lon=-100.150002, lat=-64.845955, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[559](lon=-100.050003, lat=-64.845955, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[560](lon=-100.449997, lat=-64.803413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[561](lon=-100.349998, lat=-64.803413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[562](lon=-100.250000, lat=-64.803413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[563](lon=-100.150002, lat=-64.803413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[564](lon=-100.050003, lat=-64.803413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[565](lon=-100.449997, lat=-64.760811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[566](lon=-100.349998, lat=-64.760811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[567](lon=-100.250000, lat=-64.760811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[568](lon=-100.150002, lat=-64.760811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[569](lon=-100.050003, lat=-64.760811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[570](lon=-100.449997, lat=-64.718132, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[571](lon=-100.349998, lat=-64.718132, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[572](lon=-100.250000, lat=-64.718132, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[573](lon=-100.150002, lat=-64.718132, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[574](lon=-100.050003, lat=-64.718132, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[575](lon=-100.449997, lat=-64.675392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[576](lon=-100.349998, lat=-64.675392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[577](lon=-100.250000, lat=-64.675392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[578](lon=-100.150002, lat=-64.675392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[579](lon=-100.050003, lat=-64.675392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[580](lon=-100.449997, lat=-64.632584, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[581](lon=-100.349998, lat=-64.632584, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[582](lon=-100.250000, lat=-64.632584, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[583](lon=-100.150002, lat=-64.632584, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[584](lon=-100.050003, lat=-64.632584, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[585](lon=-100.449997, lat=-64.589706, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[586](lon=-100.349998, lat=-64.589706, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[587](lon=-100.250000, lat=-64.589706, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[588](lon=-100.150002, lat=-64.589706, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[589](lon=-100.050003, lat=-64.589706, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[590](lon=-100.449997, lat=-64.546768, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[591](lon=-100.349998, lat=-64.546768, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[592](lon=-100.250000, lat=-64.546768, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[593](lon=-100.150002, lat=-64.546768, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[594](lon=-100.050003, lat=-64.546768, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[595](lon=-100.449997, lat=-64.503754, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[596](lon=-100.349998, lat=-64.503754, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[597](lon=-100.250000, lat=-64.503754, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[598](lon=-100.150002, lat=-64.503754, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[599](lon=-100.050003, lat=-64.503754, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[600](lon=-100.449997, lat=-64.460678, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[601](lon=-100.349998, lat=-64.460678, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[602](lon=-100.250000, lat=-64.460678, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[603](lon=-100.150002, lat=-64.460678, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[604](lon=-100.050003, lat=-64.460678, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[605](lon=-100.449997, lat=-64.417526, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[606](lon=-100.349998, lat=-64.417526, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[607](lon=-100.250000, lat=-64.417526, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[608](lon=-100.150002, lat=-64.417526, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[609](lon=-100.050003, lat=-64.417526, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[610](lon=-100.449997, lat=-64.374313, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[611](lon=-100.349998, lat=-64.374313, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[612](lon=-100.250000, lat=-64.374313, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[613](lon=-100.150002, lat=-64.374313, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[614](lon=-100.050003, lat=-64.374313, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[615](lon=-100.449997, lat=-64.331032, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[616](lon=-100.349998, lat=-64.331032, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[617](lon=-100.250000, lat=-64.331032, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[618](lon=-100.150002, lat=-64.331032, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[619](lon=-100.050003, lat=-64.331032, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[620](lon=-100.449997, lat=-64.287682, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[621](lon=-100.349998, lat=-64.287682, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[622](lon=-100.250000, lat=-64.287682, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[623](lon=-100.150002, lat=-64.287682, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[624](lon=-100.050003, lat=-64.287682, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[625](lon=-100.449997, lat=-64.244263, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[626](lon=-100.349998, lat=-64.244263, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[627](lon=-100.250000, lat=-64.244263, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[628](lon=-100.150002, lat=-64.244263, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[629](lon=-100.050003, lat=-64.244263, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[630](lon=-100.449997, lat=-64.200775, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[631](lon=-100.349998, lat=-64.200775, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[632](lon=-100.250000, lat=-64.200775, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[633](lon=-100.150002, lat=-64.200775, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[634](lon=-100.050003, lat=-64.200775, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[635](lon=-100.449997, lat=-64.157219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[636](lon=-100.349998, lat=-64.157219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[637](lon=-100.250000, lat=-64.157219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[638](lon=-100.150002, lat=-64.157219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[639](lon=-100.050003, lat=-64.157219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[640](lon=-100.449997, lat=-64.113594, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[641](lon=-100.349998, lat=-64.113594, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[642](lon=-100.250000, lat=-64.113594, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[643](lon=-100.150002, lat=-64.113594, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[644](lon=-100.050003, lat=-64.113594, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[645](lon=-100.449997, lat=-64.069901, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[646](lon=-100.349998, lat=-64.069901, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[647](lon=-100.250000, lat=-64.069901, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[648](lon=-100.150002, lat=-64.069901, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[649](lon=-100.050003, lat=-64.069901, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[650](lon=-100.449997, lat=-64.026138, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[651](lon=-100.349998, lat=-64.026138, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[652](lon=-100.250000, lat=-64.026138, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[653](lon=-100.150002, lat=-64.026138, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[654](lon=-100.050003, lat=-64.026138, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[655](lon=-100.449997, lat=-63.982307, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[656](lon=-100.349998, lat=-63.982307, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[657](lon=-100.250000, lat=-63.982307, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[658](lon=-100.150002, lat=-63.982307, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[659](lon=-100.050003, lat=-63.982307, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[660](lon=-100.449997, lat=-63.938408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[661](lon=-100.349998, lat=-63.938408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[662](lon=-100.250000, lat=-63.938408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[663](lon=-100.150002, lat=-63.938408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[664](lon=-100.050003, lat=-63.938408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[665](lon=-100.449997, lat=-63.894440, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[666](lon=-100.349998, lat=-63.894440, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[667](lon=-100.250000, lat=-63.894440, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[668](lon=-100.150002, lat=-63.894440, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[669](lon=-100.050003, lat=-63.894440, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[670](lon=-100.449997, lat=-63.850403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[671](lon=-100.349998, lat=-63.850403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[672](lon=-100.250000, lat=-63.850403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[673](lon=-100.150002, lat=-63.850403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[674](lon=-100.050003, lat=-63.850403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[675](lon=-100.449997, lat=-63.806293, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[676](lon=-100.349998, lat=-63.806293, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[677](lon=-100.250000, lat=-63.806293, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[678](lon=-100.150002, lat=-63.806293, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[679](lon=-100.050003, lat=-63.806293, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[680](lon=-100.449997, lat=-63.762119, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[681](lon=-100.349998, lat=-63.762119, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[682](lon=-100.250000, lat=-63.762119, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[683](lon=-100.150002, lat=-63.762119, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[684](lon=-100.050003, lat=-63.762119, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[685](lon=-100.449997, lat=-63.717876, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[686](lon=-100.349998, lat=-63.717876, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[687](lon=-100.250000, lat=-63.717876, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[688](lon=-100.150002, lat=-63.717876, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[689](lon=-100.050003, lat=-63.717876, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[690](lon=-100.449997, lat=-63.673561, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[691](lon=-100.349998, lat=-63.673561, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[692](lon=-100.250000, lat=-63.673561, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[693](lon=-100.150002, lat=-63.673561, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[694](lon=-100.050003, lat=-63.673561, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[695](lon=-100.449997, lat=-63.629177, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[696](lon=-100.349998, lat=-63.629177, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[697](lon=-100.250000, lat=-63.629177, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[698](lon=-100.150002, lat=-63.629177, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[699](lon=-100.050003, lat=-63.629177, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[700](lon=-100.449997, lat=-63.584724, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[701](lon=-100.349998, lat=-63.584724, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[702](lon=-100.250000, lat=-63.584724, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[703](lon=-100.150002, lat=-63.584724, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[704](lon=-100.050003, lat=-63.584724, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[705](lon=-100.449997, lat=-63.540203, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[706](lon=-100.349998, lat=-63.540203, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[707](lon=-100.250000, lat=-63.540203, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[708](lon=-100.150002, lat=-63.540203, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[709](lon=-100.050003, lat=-63.540203, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[710](lon=-100.449997, lat=-63.495613, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[711](lon=-100.349998, lat=-63.495613, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[712](lon=-100.250000, lat=-63.495613, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[713](lon=-100.150002, lat=-63.495613, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[714](lon=-100.050003, lat=-63.495613, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[715](lon=-100.449997, lat=-63.450951, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[716](lon=-100.349998, lat=-63.450951, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[717](lon=-100.250000, lat=-63.450951, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[718](lon=-100.150002, lat=-63.450951, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[719](lon=-100.050003, lat=-63.450951, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[720](lon=-100.449997, lat=-63.406219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[721](lon=-100.349998, lat=-63.406219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[722](lon=-100.250000, lat=-63.406219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[723](lon=-100.150002, lat=-63.406219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[724](lon=-100.050003, lat=-63.406219, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[725](lon=-100.449997, lat=-63.361420, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[726](lon=-100.349998, lat=-63.361420, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[727](lon=-100.250000, lat=-63.361420, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[728](lon=-100.150002, lat=-63.361420, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[729](lon=-100.050003, lat=-63.361420, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[730](lon=-100.449997, lat=-63.316547, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[731](lon=-100.349998, lat=-63.316547, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[732](lon=-100.250000, lat=-63.316547, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[733](lon=-100.150002, lat=-63.316547, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[734](lon=-100.050003, lat=-63.316547, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[735](lon=-100.449997, lat=-63.271606, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[736](lon=-100.349998, lat=-63.271606, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[737](lon=-100.250000, lat=-63.271606, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[738](lon=-100.150002, lat=-63.271606, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[739](lon=-100.050003, lat=-63.271606, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[740](lon=-100.449997, lat=-63.226593, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[741](lon=-100.349998, lat=-63.226593, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[742](lon=-100.250000, lat=-63.226593, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[743](lon=-100.150002, lat=-63.226593, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[744](lon=-100.050003, lat=-63.226593, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[745](lon=-100.449997, lat=-63.181515, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[746](lon=-100.349998, lat=-63.181515, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[747](lon=-100.250000, lat=-63.181515, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[748](lon=-100.150002, lat=-63.181515, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[749](lon=-100.050003, lat=-63.181515, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[750](lon=-100.449997, lat=-63.136360, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[751](lon=-100.349998, lat=-63.136360, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[752](lon=-100.250000, lat=-63.136360, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[753](lon=-100.150002, lat=-63.136360, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[754](lon=-100.050003, lat=-63.136360, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[755](lon=-100.449997, lat=-63.091141, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[756](lon=-100.349998, lat=-63.091141, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[757](lon=-100.250000, lat=-63.091141, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[758](lon=-100.150002, lat=-63.091141, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[759](lon=-100.050003, lat=-63.091141, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[760](lon=-100.449997, lat=-63.045845, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[761](lon=-100.349998, lat=-63.045845, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[762](lon=-100.250000, lat=-63.045845, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[763](lon=-100.150002, lat=-63.045845, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[764](lon=-100.050003, lat=-63.045845, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[765](lon=-100.449997, lat=-63.000484, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[766](lon=-100.349998, lat=-63.000484, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[767](lon=-100.250000, lat=-63.000484, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[768](lon=-100.150002, lat=-63.000484, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[769](lon=-100.050003, lat=-63.000484, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[770](lon=-100.449997, lat=-62.955051, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[771](lon=-100.349998, lat=-62.955051, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[772](lon=-100.250000, lat=-62.955051, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[773](lon=-100.150002, lat=-62.955051, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[774](lon=-100.050003, lat=-62.955051, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[775](lon=-100.449997, lat=-62.909546, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[776](lon=-100.349998, lat=-62.909546, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[777](lon=-100.250000, lat=-62.909546, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[778](lon=-100.150002, lat=-62.909546, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[779](lon=-100.050003, lat=-62.909546, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[780](lon=-100.449997, lat=-62.863972, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[781](lon=-100.349998, lat=-62.863972, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[782](lon=-100.250000, lat=-62.863972, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[783](lon=-100.150002, lat=-62.863972, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[784](lon=-100.050003, lat=-62.863972, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[785](lon=-100.449997, lat=-62.818325, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[786](lon=-100.349998, lat=-62.818325, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[787](lon=-100.250000, lat=-62.818325, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[788](lon=-100.150002, lat=-62.818325, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[789](lon=-100.050003, lat=-62.818325, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[790](lon=-100.449997, lat=-62.772610, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[791](lon=-100.349998, lat=-62.772610, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[792](lon=-100.250000, lat=-62.772610, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[793](lon=-100.150002, lat=-62.772610, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[794](lon=-100.050003, lat=-62.772610, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[795](lon=-100.449997, lat=-62.726822, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[796](lon=-100.349998, lat=-62.726822, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[797](lon=-100.250000, lat=-62.726822, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[798](lon=-100.150002, lat=-62.726822, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[799](lon=-100.050003, lat=-62.726822, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[800](lon=-100.449997, lat=-62.680962, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[801](lon=-100.349998, lat=-62.680962, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[802](lon=-100.250000, lat=-62.680962, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[803](lon=-100.150002, lat=-62.680962, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[804](lon=-100.050003, lat=-62.680962, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[805](lon=-100.449997, lat=-62.635033, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[806](lon=-100.349998, lat=-62.635033, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[807](lon=-100.250000, lat=-62.635033, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[808](lon=-100.150002, lat=-62.635033, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[809](lon=-100.050003, lat=-62.635033, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[810](lon=-100.449997, lat=-62.589031, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[811](lon=-100.349998, lat=-62.589031, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[812](lon=-100.250000, lat=-62.589031, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[813](lon=-100.150002, lat=-62.589031, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[814](lon=-100.050003, lat=-62.589031, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[815](lon=-100.449997, lat=-62.542957, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[816](lon=-100.349998, lat=-62.542957, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[817](lon=-100.250000, lat=-62.542957, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[818](lon=-100.150002, lat=-62.542957, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[819](lon=-100.050003, lat=-62.542957, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[820](lon=-100.449997, lat=-62.496815, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[821](lon=-100.349998, lat=-62.496815, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[822](lon=-100.250000, lat=-62.496815, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[823](lon=-100.150002, lat=-62.496815, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[824](lon=-100.050003, lat=-62.496815, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[825](lon=-100.449997, lat=-62.450600, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[826](lon=-100.349998, lat=-62.450600, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[827](lon=-100.250000, lat=-62.450600, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[828](lon=-100.150002, lat=-62.450600, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[829](lon=-100.050003, lat=-62.450600, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[830](lon=-100.449997, lat=-62.404312, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[831](lon=-100.349998, lat=-62.404312, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[832](lon=-100.250000, lat=-62.404312, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[833](lon=-100.150002, lat=-62.404312, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[834](lon=-100.050003, lat=-62.404312, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[835](lon=-100.449997, lat=-62.357952, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[836](lon=-100.349998, lat=-62.357952, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[837](lon=-100.250000, lat=-62.357952, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[838](lon=-100.150002, lat=-62.357952, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[839](lon=-100.050003, lat=-62.357952, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[840](lon=-100.449997, lat=-62.311520, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[841](lon=-100.349998, lat=-62.311520, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[842](lon=-100.250000, lat=-62.311520, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[843](lon=-100.150002, lat=-62.311520, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[844](lon=-100.050003, lat=-62.311520, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[845](lon=-100.449997, lat=-62.265018, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[846](lon=-100.349998, lat=-62.265018, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[847](lon=-100.250000, lat=-62.265018, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[848](lon=-100.150002, lat=-62.265018, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[849](lon=-100.050003, lat=-62.265018, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[850](lon=-100.449997, lat=-62.218445, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[851](lon=-100.349998, lat=-62.218445, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[852](lon=-100.250000, lat=-62.218445, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[853](lon=-100.150002, lat=-62.218445, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[854](lon=-100.050003, lat=-62.218445, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[855](lon=-100.449997, lat=-62.171799, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[856](lon=-100.349998, lat=-62.171799, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[857](lon=-100.250000, lat=-62.171799, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[858](lon=-100.150002, lat=-62.171799, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[859](lon=-100.050003, lat=-62.171799, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[860](lon=-100.449997, lat=-62.125080, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[861](lon=-100.349998, lat=-62.125080, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[862](lon=-100.250000, lat=-62.125080, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[863](lon=-100.150002, lat=-62.125080, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[864](lon=-100.050003, lat=-62.125080, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[865](lon=-100.449997, lat=-62.078289, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[866](lon=-100.349998, lat=-62.078289, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[867](lon=-100.250000, lat=-62.078289, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[868](lon=-100.150002, lat=-62.078289, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[869](lon=-100.050003, lat=-62.078289, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[870](lon=-100.449997, lat=-62.031429, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[871](lon=-100.349998, lat=-62.031429, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[872](lon=-100.250000, lat=-62.031429, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[873](lon=-100.150002, lat=-62.031429, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[874](lon=-100.050003, lat=-62.031429, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[875](lon=-100.449997, lat=-61.984493, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[876](lon=-100.349998, lat=-61.984493, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[877](lon=-100.250000, lat=-61.984493, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[878](lon=-100.150002, lat=-61.984493, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[879](lon=-100.050003, lat=-61.984493, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[880](lon=-100.449997, lat=-61.937485, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[881](lon=-100.349998, lat=-61.937485, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[882](lon=-100.250000, lat=-61.937485, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[883](lon=-100.150002, lat=-61.937485, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[884](lon=-100.050003, lat=-61.937485, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[885](lon=-100.449997, lat=-61.890408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[886](lon=-100.349998, lat=-61.890408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[887](lon=-100.250000, lat=-61.890408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[888](lon=-100.150002, lat=-61.890408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[889](lon=-100.050003, lat=-61.890408, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[890](lon=-100.449997, lat=-61.843254, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[891](lon=-100.349998, lat=-61.843254, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[892](lon=-100.250000, lat=-61.843254, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[893](lon=-100.150002, lat=-61.843254, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[894](lon=-100.050003, lat=-61.843254, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[895](lon=-100.449997, lat=-61.796028, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[896](lon=-100.349998, lat=-61.796028, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[897](lon=-100.250000, lat=-61.796028, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[898](lon=-100.150002, lat=-61.796028, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[899](lon=-100.050003, lat=-61.796028, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[900](lon=-100.449997, lat=-61.748730, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[901](lon=-100.349998, lat=-61.748730, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[902](lon=-100.250000, lat=-61.748730, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[903](lon=-100.150002, lat=-61.748730, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[904](lon=-100.050003, lat=-61.748730, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[905](lon=-100.449997, lat=-61.701363, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[906](lon=-100.349998, lat=-61.701363, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[907](lon=-100.250000, lat=-61.701363, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[908](lon=-100.150002, lat=-61.701363, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[909](lon=-100.050003, lat=-61.701363, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[910](lon=-100.449997, lat=-61.653919, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[911](lon=-100.349998, lat=-61.653919, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[912](lon=-100.250000, lat=-61.653919, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[913](lon=-100.150002, lat=-61.653919, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[914](lon=-100.050003, lat=-61.653919, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[915](lon=-100.449997, lat=-61.606403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[916](lon=-100.349998, lat=-61.606403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[917](lon=-100.250000, lat=-61.606403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[918](lon=-100.150002, lat=-61.606403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[919](lon=-100.050003, lat=-61.606403, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[920](lon=-100.449997, lat=-61.558811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[921](lon=-100.349998, lat=-61.558811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[922](lon=-100.250000, lat=-61.558811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[923](lon=-100.150002, lat=-61.558811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[924](lon=-100.050003, lat=-61.558811, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[925](lon=-100.449997, lat=-61.511150, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[926](lon=-100.349998, lat=-61.511150, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[927](lon=-100.250000, lat=-61.511150, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[928](lon=-100.150002, lat=-61.511150, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[929](lon=-100.050003, lat=-61.511150, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[930](lon=-100.449997, lat=-61.463413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[931](lon=-100.349998, lat=-61.463413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[932](lon=-100.250000, lat=-61.463413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[933](lon=-100.150002, lat=-61.463413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[934](lon=-100.050003, lat=-61.463413, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[935](lon=-100.449997, lat=-61.415607, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[936](lon=-100.349998, lat=-61.415607, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[937](lon=-100.250000, lat=-61.415607, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[938](lon=-100.150002, lat=-61.415607, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[939](lon=-100.050003, lat=-61.415607, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[940](lon=-100.449997, lat=-61.367725, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[941](lon=-100.349998, lat=-61.367725, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[942](lon=-100.250000, lat=-61.367725, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[943](lon=-100.150002, lat=-61.367725, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[944](lon=-100.050003, lat=-61.367725, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[945](lon=-100.449997, lat=-61.319771, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[946](lon=-100.349998, lat=-61.319771, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[947](lon=-100.250000, lat=-61.319771, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[948](lon=-100.150002, lat=-61.319771, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[949](lon=-100.050003, lat=-61.319771, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[950](lon=-100.449997, lat=-61.271740, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[951](lon=-100.349998, lat=-61.271740, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[952](lon=-100.250000, lat=-61.271740, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[953](lon=-100.150002, lat=-61.271740, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[954](lon=-100.050003, lat=-61.271740, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[955](lon=-100.449997, lat=-61.223637, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[956](lon=-100.349998, lat=-61.223637, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[957](lon=-100.250000, lat=-61.223637, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[958](lon=-100.150002, lat=-61.223637, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[959](lon=-100.050003, lat=-61.223637, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[960](lon=-100.449997, lat=-61.175461, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[961](lon=-100.349998, lat=-61.175461, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[962](lon=-100.250000, lat=-61.175461, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[963](lon=-100.150002, lat=-61.175461, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[964](lon=-100.050003, lat=-61.175461, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[965](lon=-100.449997, lat=-61.127213, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[966](lon=-100.349998, lat=-61.127213, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[967](lon=-100.250000, lat=-61.127213, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[968](lon=-100.150002, lat=-61.127213, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[969](lon=-100.050003, lat=-61.127213, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[970](lon=-100.449997, lat=-61.078888, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[971](lon=-100.349998, lat=-61.078888, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[972](lon=-100.250000, lat=-61.078888, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[973](lon=-100.150002, lat=-61.078888, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[974](lon=-100.050003, lat=-61.078888, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[975](lon=-100.449997, lat=-61.030491, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[976](lon=-100.349998, lat=-61.030491, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[977](lon=-100.250000, lat=-61.030491, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[978](lon=-100.150002, lat=-61.030491, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[979](lon=-100.050003, lat=-61.030491, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[980](lon=-100.449997, lat=-60.982021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[981](lon=-100.349998, lat=-60.982021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[982](lon=-100.250000, lat=-60.982021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[983](lon=-100.150002, lat=-60.982021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[984](lon=-100.050003, lat=-60.982021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[985](lon=-100.449997, lat=-60.933475, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[986](lon=-100.349998, lat=-60.933475, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[987](lon=-100.250000, lat=-60.933475, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[988](lon=-100.150002, lat=-60.933475, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[989](lon=-100.050003, lat=-60.933475, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[990](lon=-100.449997, lat=-60.884853, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[991](lon=-100.349998, lat=-60.884853, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[992](lon=-100.250000, lat=-60.884853, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[993](lon=-100.150002, lat=-60.884853, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[994](lon=-100.050003, lat=-60.884853, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[995](lon=-100.449997, lat=-60.836163, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[996](lon=-100.349998, lat=-60.836163, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[997](lon=-100.250000, lat=-60.836163, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[998](lon=-100.150002, lat=-60.836163, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[999](lon=-100.050003, lat=-60.836163, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1000](lon=-100.449997, lat=-60.787392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1001](lon=-100.349998, lat=-60.787392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1002](lon=-100.250000, lat=-60.787392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1003](lon=-100.150002, lat=-60.787392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1004](lon=-100.050003, lat=-60.787392, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1005](lon=-100.449997, lat=-60.738552, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1006](lon=-100.349998, lat=-60.738552, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1007](lon=-100.250000, lat=-60.738552, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1008](lon=-100.150002, lat=-60.738552, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1009](lon=-100.050003, lat=-60.738552, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1010](lon=-100.449997, lat=-60.689632, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1011](lon=-100.349998, lat=-60.689632, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1012](lon=-100.250000, lat=-60.689632, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1013](lon=-100.150002, lat=-60.689632, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1014](lon=-100.050003, lat=-60.689632, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1015](lon=-100.449997, lat=-60.640644, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1016](lon=-100.349998, lat=-60.640644, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1017](lon=-100.250000, lat=-60.640644, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1018](lon=-100.150002, lat=-60.640644, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1019](lon=-100.050003, lat=-60.640644, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1020](lon=-100.449997, lat=-60.591576, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1021](lon=-100.349998, lat=-60.591576, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1022](lon=-100.250000, lat=-60.591576, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1023](lon=-100.150002, lat=-60.591576, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1024](lon=-100.050003, lat=-60.591576, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1025](lon=-100.449997, lat=-60.542435, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1026](lon=-100.349998, lat=-60.542435, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1027](lon=-100.250000, lat=-60.542435, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1028](lon=-100.150002, lat=-60.542435, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1029](lon=-100.050003, lat=-60.542435, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1030](lon=-100.449997, lat=-60.493221, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1031](lon=-100.349998, lat=-60.493221, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1032](lon=-100.250000, lat=-60.493221, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1033](lon=-100.150002, lat=-60.493221, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1034](lon=-100.050003, lat=-60.493221, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1035](lon=-100.449997, lat=-60.443932, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1036](lon=-100.349998, lat=-60.443932, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1037](lon=-100.250000, lat=-60.443932, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1038](lon=-100.150002, lat=-60.443932, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1039](lon=-100.050003, lat=-60.443932, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1040](lon=-100.449997, lat=-60.394566, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1041](lon=-100.349998, lat=-60.394566, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1042](lon=-100.250000, lat=-60.394566, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1043](lon=-100.150002, lat=-60.394566, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1044](lon=-100.050003, lat=-60.394566, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1045](lon=-100.449997, lat=-60.345127, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1046](lon=-100.349998, lat=-60.345127, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1047](lon=-100.250000, lat=-60.345127, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1048](lon=-100.150002, lat=-60.345127, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1049](lon=-100.050003, lat=-60.345127, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1050](lon=-100.449997, lat=-60.295612, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1051](lon=-100.349998, lat=-60.295612, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1052](lon=-100.250000, lat=-60.295612, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1053](lon=-100.150002, lat=-60.295612, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1054](lon=-100.050003, lat=-60.295612, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1055](lon=-100.449997, lat=-60.246021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1056](lon=-100.349998, lat=-60.246021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1057](lon=-100.250000, lat=-60.246021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1058](lon=-100.150002, lat=-60.246021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1059](lon=-100.050003, lat=-60.246021, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1060](lon=-100.449997, lat=-60.196358, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1061](lon=-100.349998, lat=-60.196358, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1062](lon=-100.250000, lat=-60.196358, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1063](lon=-100.150002, lat=-60.196358, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1064](lon=-100.050003, lat=-60.196358, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1065](lon=-100.449997, lat=-60.146614, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1066](lon=-100.349998, lat=-60.146614, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1067](lon=-100.250000, lat=-60.146614, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1068](lon=-100.150002, lat=-60.146614, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1069](lon=-100.050003, lat=-60.146614, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1070](lon=-100.449997, lat=-60.096798, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1071](lon=-100.349998, lat=-60.096798, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1072](lon=-100.250000, lat=-60.096798, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1073](lon=-100.150002, lat=-60.096798, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1074](lon=-100.050003, lat=-60.096798, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1075](lon=-100.449997, lat=-60.046909, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1076](lon=-100.349998, lat=-60.046909, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1077](lon=-100.250000, lat=-60.046909, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1078](lon=-100.150002, lat=-60.046909, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
P[1079](lon=-100.050003, lat=-60.046909, depth=10.000000, thermo=0.000000, psal=0.000000, time=0.000000)
For the 3D advection we will integrate particles forward-in-time for 20 days to keep this example simple, but feel free to integrate longer! If you want really long integrations you should consider submitting the analysis as a job on Gadi or to some other HPC system of your liking.
Note 1: Generally you want to use a small integration timestep (dt
). This is because, the larger the dt
, the further particles will move in a single timestep and the more likely you are to get particles crossing ocean-land boundaries and ‘beaching’. For example, in some Lagrangian applications it is common to use dt
<= 15 minutes. However the choice of dt
has to be balanced against the computational resources and the available simulation time. In this example, we’ll use a
dt
of 2 hours to speed up the integration. If you want to integrate particles backwards-in-time, simple feed Parcels a negative dt
value.
Note 2: This 3D example may take some time (~5-10 minutes) depending on the resources you’re using.
[20]:
# Set your output location:
output_filename = 'TestParticles_3D.zarr'
# the file name and the time step of the outputs
# (particle locations will be saved every 5 days in this example)
output_file = pset.ParticleFile(name=output_directory + output_filename,
outputdt=timedelta(days=2))
pset.execute(
[
AdvectionRK4_3D, # the 3D advection kernel (which defines how particles move)
periodicBC,
SampleFields,
checkOutOfBounds
],
runtime=timedelta(days=20), # the total length of the run
dt=timedelta(hours=2), # the integration timestep of the kernel
output_file=output_file, # the output file
)
INFO: Output files are stored in /scratch/tm70/as2285/particle_trackingTestParticles_3D.zarr.
100%|██████████| 1728000.0/1728000.0 [01:16<00:00, 22515.87it/s]
Open output file and scatter plot the first 100 of these trajectories, coloured by depth.
[21]:
ds = xr.open_zarr(output_directory + output_filename)
fig = plt.figure(figsize=(12, 8))
ht.plot(cmap='Blues')
plt.plot(ds.lon[:100, :].T, ds.lat[:100, :].T, c='w', zorder=2)
cb = plt.scatter(ds.lon[:100, 1:], ds.lat[:100, 1:], c=ds.z[:100, 1:],
s=20, vmin=3, vmax=18, cmap='Reds', zorder=3)
plt.legend(*cb.legend_elements(num=10), loc="upper right", title="Depth (m)")
plt.ylim([-68, -62])
plt.xlim([-108, -95]);
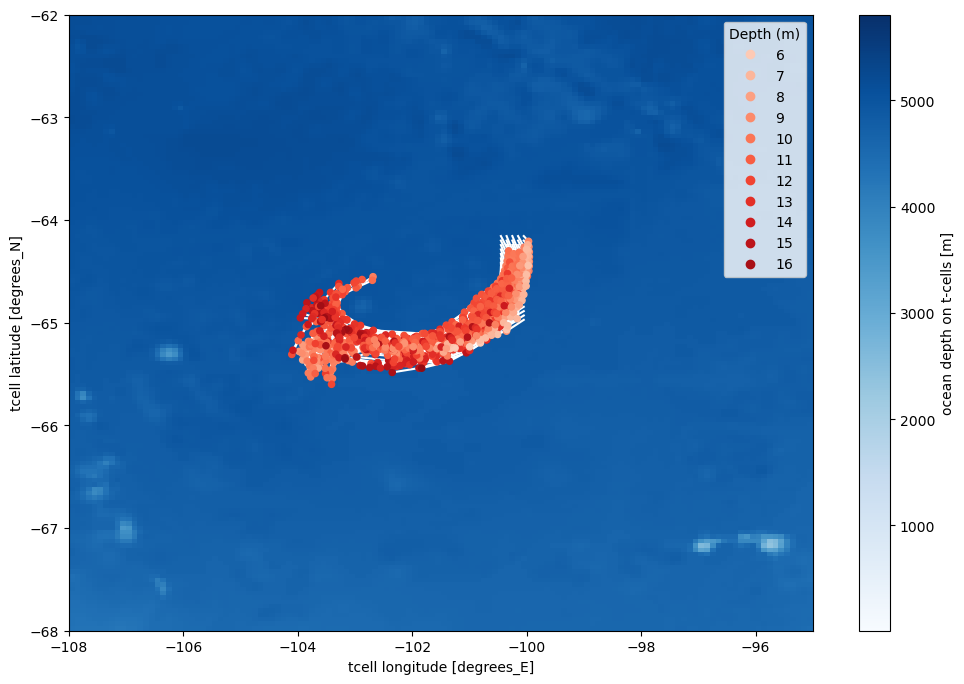
For reference, this is how the output file is organised.
[22]:
ds
[22]:
<xarray.Dataset> Dimensions: (trajectory: 540, obs: 10) Coordinates: * obs (obs) int32 0 1 2 3 4 5 6 7 8 9 * trajectory (trajectory) int64 540 541 542 543 544 ... 1076 1077 1078 1079 Data variables: lat (trajectory, obs) float32 dask.array<chunksize=(540, 1), meta=np.ndarray> lon (trajectory, obs) float32 dask.array<chunksize=(540, 1), meta=np.ndarray> psal (trajectory, obs) float32 dask.array<chunksize=(540, 1), meta=np.ndarray> thermo (trajectory, obs) float32 dask.array<chunksize=(540, 1), meta=np.ndarray> time (trajectory, obs) object dask.array<chunksize=(540, 1), meta=np.ndarray> z (trajectory, obs) float32 dask.array<chunksize=(540, 1), meta=np.ndarray> Attributes: Conventions: CF-1.6/CF-1.7 feature_type: trajectory ncei_template_version: NCEI_NetCDF_Trajectory_Template_v2.0 parcels_kernels: SampleParticleAdvectionRK4_3DperiodicBCSampleFiel... parcels_mesh: spherical parcels_version: 3.0.0
[23]:
client.close()